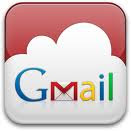
In this post we will learn how to send attachment in email like file or image also how to embed the image inside the email.
The good thing about Gmail is that we can use it to send emails as SMTP server.
Here is the needed steps:
1.Define the following attributes:
smtpServerIP = smtp.gmail.com;
smtpServerPort = 465;
smtpServerUser = Your gmail email;
smtpServerPassword = Your gmail email password;
2.The following is mail sending method:
public void sendMail(final String[] toUserEmail, final String subject, final String body,final BufferedImage myImage, final File file, final boolean isPicture) {
new Thread() {
@Override
public void run() {
try {
Properties props = new Properties();
props.put("mail.smtp.host", smtpServerIP);
props.put("mail.smtp.port", smtpServerPort);
props.put("mail.smtp.auth", "true");
//props.put("mail.debug", "false");
props.put("mail.smtp.socketFactory.port", smtpServerPort);
props.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory");
props.put("mail.smtp.socketFactory.fallback", "false");
Authenticator auth = new SMTPAuthenticator();
Session session = Session.getInstance(props, auth);
//session.setDebug(false);
// create a message
Message msg = new MimeMessage(session);
msg.setHeader("HomeMonitor", "By Osama Oransa");
// set the from and to address
InternetAddress addressFrom = new InternetAddress(smtpServerUser);
msg.setFrom(addressFrom);
InternetAddress[] addressTo = new InternetAddress[toUserEmail.length];
for (int i = 0; i < toUserEmail.length; i++) {
addressTo[i] = new InternetAddress(toUserEmail[i]);
}
msg.setRecipients(Message.RecipientType.TO, addressTo);
String[] messageParts = body.split("##");
//no thing attached
if (messageParts.length == 1) {
msg.setContent(body, "text/html");
//image is attached
//body is text and image
} else if (messageParts.length == 2) {
MimeMultipart multipart = new MimeMultipart();//"related");
MimeBodyPart messageBodyPart;
// first part html text before image
messageBodyPart = new MimeBodyPart();
if (isPicture) {
messageBodyPart.setContent(messageParts[0] + "
" + messageParts[1], "text/html");
multipart.addBodyPart(messageBodyPart);
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
boolean readingImageResult;
try {
readingImageResult = ImageIO.write(myImage, "jpg", byteArrayOutputStream);
System.out.println("Result of writing the image is " + readingImageResult);
} catch (IOException ex) {
ex.printStackTrace();
}
byte[] byteArray = byteArrayOutputStream.toByteArray();
MimeBodyPart messageBodyPart2 = new MimeBodyPart();
DataSource fds = new ByteArrayDataSource(byteArray, "image/jpg");
messageBodyPart2.setDataHandler(new DataHandler(fds));
messageBodyPart2.setHeader("Content-ID", "
multipart.addBodyPart(messageBodyPart2);
msg.setContent(multipart);
} else {
//is file attached, like voice....
messageBodyPart.setContent(messageParts[0] + "
" + messageParts[1], "text/html");
multipart.addBodyPart(messageBodyPart);
// Part two is attachment
MimeBodyPart messageBodyPart2 = new MimeBodyPart();
DataSource source = new FileDataSource(file);
messageBodyPart2.setDataHandler(new DataHandler(source));
messageBodyPart2.setFileName(file.getName());
multipart.addBodyPart(messageBodyPart2);
msg.setContent(multipart);
}
}
// Setting the Subject and Content Type
msg.setSubject(subject);
Transport.send(msg);
System.out.println("Message sent to " + toUserEmail + " OK.");
} catch (Exception ex) {
ex.printStackTrace();
System.out.println("Exception " + ex);
}
}
}.start();
}
3. Define inner class for authentication:
// Also include an inner class that is used for authentication purposes
private class SMTPAuthenticator extends javax.mail.Authenticator {
@Override
public PasswordAuthentication getPasswordAuthentication() {
System.out.println("call authentication");
String username = smtpServerUser;
String password = smtpServerPassword;
return new PasswordAuthentication(username, password);
}
}
The method send email either plain email or with attachement or with embeded picture in it.
final String[] toUserEmail, final String subject, final String body,final BufferedImage myImage, final File file, final boolean isPicture)
It takes array of emails to send to them, subject and body of the email.
Then it take optional image or file to attach (not both so you can modify the method to include this extra-requirements)
The boolean isPicture is used to deal with picture as embedded object..
In case you need to attach image or file , you need to place in the body of the email the token ## file name or image name (or title).
This is easy usage method for sending emails with attachment and in our example we used Gmail as SMTP server.
No comments:
Post a Comment