In this post we will show how to use a USB camera to capture a picture from inside a Java application..
We will use the library LTI-CIVIL which is part of FMJ (Freedom for Media in Java)
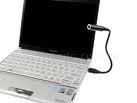
Let's go through the needed steps:
1.Download LTI-CIVIL:
Which is a Java library for capturing images from a video source such as a USB camera. It provides a simple API and does not depend on or use JMF!
The advantage behind this library that it doesn't depend on JMF (which is almost very old now)
The project URL: http://lti-civil.org/
Download URL: http://sourceforge.net/projects/lti-civil/files/
Extract the zip/tar file and you will find 2 things we will use:
a) lti-civil-no_s_w_t.jar
b) native folder contain the native libraries according to the operating system , pick the one for the system you are using.
2.Create the Java App:
Add to the class-path of the project the jar file and the native library folder.
To Run the project as jar file , you can use the JVM parameter:
-Djava.library.path=".....\native\win32-x86" to add the library to the class path of the jar file.
3.Create a class file:
Name it like TestWebCam implements CaptureObserver
4. Define the following instance variables:
JButton start = null;
JButton shot = null;
JButton stop = null;
CaptureStream captureStream = null;
boolean takeShot=false;
5.In the constructor:
Add the following code to initialize the capturing device:
public TestWebCam() {
CaptureSystemFactory factory = DefaultCaptureSystemFactorySingleton.instance();
CaptureSystem system;
try {
system = factory.createCaptureSystem();
system.init();
List list = system.getCaptureDeviceInfoList();
int i = 0;
if (i < list.size()) {
CaptureDeviceInfo info = (CaptureDeviceInfo) list.get(i);
System.out.println((new StringBuilder()).append("Device ID ").append(i).append(": ").append(info.getDeviceID()).toString());
System.out.println((new StringBuilder()).append("Description ").append(i).append(": ").append(info.getDescription()).toString());
captureStream = system.openCaptureDeviceStream(info.getDeviceID());
captureStream.setObserver(TestWebCam.this);
}
} catch (CaptureException ex) {
ex.printStackTrace();
}
//UI work of the program
JFrame frame = new JFrame();
frame.setSize(7000, 800);
JPanel panel = new JPanel();
frame.setContentPane(panel);
frame.setVisible(true);
frame.setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
start = new JButton("Start");
stop = new JButton("Stop");
shot = new JButton("Shot");
panel.add(start);
panel.add(stop);
panel.add(shot);
panel.revalidate();
start.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
captureStream.start();
} catch (CaptureException ex) {
ex.printStackTrace();
}
}
});
stop.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
captureStream.stop();
} catch (CaptureException ex) {
ex.printStackTrace();
}
}
});
shot.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
takeShot=true;
}
});
}
6.Add the following 2 methods mandated by the implemented interface CaptureObserver:
public void onNewImage(CaptureStream stream, Image image) {
if(!takeShot) return;
takeShot=false;
System.out.println("New Image Captured");
byte bytes[] = null;
try {
if (image == null) {
bytes = null;
return;
}
try {
ByteArrayOutputStream os = new ByteArrayOutputStream();
JPEGImageEncoder jpeg = JPEGCodec.createJPEGEncoder(os);
jpeg.encode(AWTImageConverter.toBufferedImage(image));
os.close();
bytes = os.toByteArray();
} catch (IOException e) {
e.printStackTrace();
bytes = null;
} catch (Throwable t) {
t.printStackTrace();
bytes = null;
}
if (bytes == null) {
return;
}
ByteArrayInputStream is = new ByteArrayInputStream(bytes);
File file = new File("/img" + Calendar.getInstance().getTimeInMillis() + ".jpg");
FileOutputStream fos = new FileOutputStream(file);
fos.write(bytes);
fos.close();
BufferedImage myImage = ImageIO.read(file);
shot.setText("");
shot.setIcon(new ImageIcon(myImage));
shot.revalidate();
} catch (IOException ex) {
ex.printStackTrace();
}
}
public void onError(CaptureStream stream, CaptureException ce) {
System.out.println("Error!");
}
7.Optionally, to Covert image to Gray Scale:
In case you need to convert it into Gray scale (for image processing purposes you can replace the following line with this block:
Old Line:
shot.setIcon(new ImageIcon(myImage));
New Block:
//Convert myImage to gray scale
BufferedImage imageGray = new BufferedImage(myImage.getWidth(), myImage.getHeight(),
BufferedImage.TYPE_BYTE_GRAY);
Graphics g = imageGray.getGraphics();
g.drawImage(myImage, 0, 0, null);
g.dispose();
shot.setIcon(new ImageIcon(imageGray));
8.Add main method to this class to run it:
public static void main(String args[])
throws Exception {
TestWebCam test = new TestWebCam();
}
9.Connect the USB Camera and run the application...
It should show 3 buttons : Start , Stop and Shot..
Click on start , then on shot, it will get you the current web camera image, move the web camera and take another shot by click on the picture to update it.
**Possible Issues:
1.Native library is not in the classpath.
2.Wrong native library is used.
3.USB Camera is not correct connected or installed.
4.Another program is running connected to the camera resource (you may be running your program 2 times)
You can use the CaptureStream parameter as well, but i think if you need to capture a streaming web cam , it would better to use images with intervals.
*** UPDATE: based on a lot of requests asking about using it to capture videos, here is the new post discussing the topic in details:
WebCam Video Capture in Java
Hope this help more to address this issue in particular.
*** UPDATE|: based on number of requests to access the web camera from JSP file , here is a link to a post discussing the how to in details:
Accessing Webcam Within JSP
*** UPDATE: if you want sample code , please download my other open source project Interactive4J, it has example for video and images.
We will use the library LTI-CIVIL which is part of FMJ (Freedom for Media in Java)
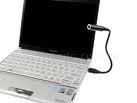
Let's go through the needed steps:
1.Download LTI-CIVIL:
Which is a Java library for capturing images from a video source such as a USB camera. It provides a simple API and does not depend on or use JMF!
The advantage behind this library that it doesn't depend on JMF (which is almost very old now)
The project URL: http://lti-civil.org/
Download URL: http://sourceforge.net/projects/lti-civil/files/
Extract the zip/tar file and you will find 2 things we will use:
a) lti-civil-no_s_w_t.jar
b) native folder contain the native libraries according to the operating system , pick the one for the system you are using.
2.Create the Java App:
Add to the class-path of the project the jar file and the native library folder.
To Run the project as jar file , you can use the JVM parameter:
-Djava.library.path=".....\native\win32-x86" to add the library to the class path of the jar file.
3.Create a class file:
Name it like TestWebCam implements CaptureObserver
4. Define the following instance variables:
JButton start = null;
JButton shot = null;
JButton stop = null;
CaptureStream captureStream = null;
boolean takeShot=false;
5.In the constructor:
Add the following code to initialize the capturing device:
public TestWebCam() {
CaptureSystemFactory factory = DefaultCaptureSystemFactorySingleton.instance();
CaptureSystem system;
try {
system = factory.createCaptureSystem();
system.init();
List list = system.getCaptureDeviceInfoList();
int i = 0;
if (i < list.size()) {
CaptureDeviceInfo info = (CaptureDeviceInfo) list.get(i);
System.out.println((new StringBuilder()).append("Device ID ").append(i).append(": ").append(info.getDeviceID()).toString());
System.out.println((new StringBuilder()).append("Description ").append(i).append(": ").append(info.getDescription()).toString());
captureStream = system.openCaptureDeviceStream(info.getDeviceID());
captureStream.setObserver(TestWebCam.this);
}
} catch (CaptureException ex) {
ex.printStackTrace();
}
//UI work of the program
JFrame frame = new JFrame();
frame.setSize(7000, 800);
JPanel panel = new JPanel();
frame.setContentPane(panel);
frame.setVisible(true);
frame.setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
start = new JButton("Start");
stop = new JButton("Stop");
shot = new JButton("Shot");
panel.add(start);
panel.add(stop);
panel.add(shot);
panel.revalidate();
start.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
captureStream.start();
} catch (CaptureException ex) {
ex.printStackTrace();
}
}
});
stop.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
captureStream.stop();
} catch (CaptureException ex) {
ex.printStackTrace();
}
}
});
shot.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
takeShot=true;
}
});
}
6.Add the following 2 methods mandated by the implemented interface CaptureObserver:
public void onNewImage(CaptureStream stream, Image image) {
if(!takeShot) return;
takeShot=false;
System.out.println("New Image Captured");
byte bytes[] = null;
try {
if (image == null) {
bytes = null;
return;
}
try {
ByteArrayOutputStream os = new ByteArrayOutputStream();
JPEGImageEncoder jpeg = JPEGCodec.createJPEGEncoder(os);
jpeg.encode(AWTImageConverter.toBufferedImage(image));
os.close();
bytes = os.toByteArray();
} catch (IOException e) {
e.printStackTrace();
bytes = null;
} catch (Throwable t) {
t.printStackTrace();
bytes = null;
}
if (bytes == null) {
return;
}
ByteArrayInputStream is = new ByteArrayInputStream(bytes);
File file = new File("/img" + Calendar.getInstance().getTimeInMillis() + ".jpg");
FileOutputStream fos = new FileOutputStream(file);
fos.write(bytes);
fos.close();
BufferedImage myImage = ImageIO.read(file);
shot.setText("");
shot.setIcon(new ImageIcon(myImage));
shot.revalidate();
} catch (IOException ex) {
ex.printStackTrace();
}
}
public void onError(CaptureStream stream, CaptureException ce) {
System.out.println("Error!");
}
7.Optionally, to Covert image to Gray Scale:
In case you need to convert it into Gray scale (for image processing purposes you can replace the following line with this block:
Old Line:
shot.setIcon(new ImageIcon(myImage));
New Block:
//Convert myImage to gray scale
BufferedImage imageGray = new BufferedImage(myImage.getWidth(), myImage.getHeight(),
BufferedImage.TYPE_BYTE_GRAY);
Graphics g = imageGray.getGraphics();
g.drawImage(myImage, 0, 0, null);
g.dispose();
shot.setIcon(new ImageIcon(imageGray));
8.Add main method to this class to run it:
public static void main(String args[])
throws Exception {
TestWebCam test = new TestWebCam();
}
9.Connect the USB Camera and run the application...
It should show 3 buttons : Start , Stop and Shot..
Click on start , then on shot, it will get you the current web camera image, move the web camera and take another shot by click on the picture to update it.
**Possible Issues:
1.Native library is not in the classpath.
2.Wrong native library is used.
3.USB Camera is not correct connected or installed.
4.Another program is running connected to the camera resource (you may be running your program 2 times)
You can use the CaptureStream parameter as well, but i think if you need to capture a streaming web cam , it would better to use images with intervals.
*** UPDATE: based on a lot of requests asking about using it to capture videos, here is the new post discussing the topic in details:
WebCam Video Capture in Java
Hope this help more to address this issue in particular.
*** UPDATE|: based on number of requests to access the web camera from JSP file , here is a link to a post discussing the how to in details:
Accessing Webcam Within JSP
*** UPDATE: if you want sample code , please download my other open source project Interactive4J, it has example for video and images.
heei thanks for this tutorial.
ReplyDeleteBut I have a problem with the folowing line:
JPEGImageEncoder jpeg = JPEGCodec.createJPEGEncoder(os);
my eclipse says: "JPEGImageEncoder cannot be resolved to a type"
do you have a solution for this problem?
thanks and greetings
Pundidas
Ensure you are using Java 5 and Sun JDK.
ReplyDeleteOr replace it with the code used in post http://osama-oransa.blogspot.com/2010/12/using-gmail-to-send-emails-with.html to send jpg in email, it highlight how to construct JPG.
import com.sun.image.codec.jpeg.*;
Deleteand add rt.jar to your project from Jre's lib folder
Harry :)
thanks for answering.
ReplyDeleteit works with Java 5 but im normally using Java 6, so I'll try to build it in Java 6. The issue is to change the JPEGImageEncoder thing, due to it dosn't exists in Java 6 I think.
Hi,
ReplyDeleteFirst of all thank you very much for providing such a typical solution.
I am using fedora-core-12 64bit and set the path like
CLASSPATH=/home/guest/Downloads/webcam/lti-civil-20070920-1721/lti-civil-no_s_w_t.jar:$CLASSPATH
LD_LIBRARY_PATH=/home/guest/Downloads/webcam/lti-civil-20070920-1721/native/linux-x86:/home/guest/Downloads/webcam/lti-civil-20070920-1721/native/linux-amd64:$LD_LIBRARY_PATH
but when i am running this sample i got an error message like :
Exception in thread "main" java.lang.NoClassDefFoundError: TestWebCam
Caused by: java.lang.ClassNotFoundException: TestWebCam
at java.net.URLClassLoader$1.run(URLClassLoader.java:202)
at java.security.AccessController.doPrivileged(Native Method)
at java.net.URLClassLoader.findClass(URLClassLoader.java:190)
at java.lang.ClassLoader.loadClass(ClassLoader.java:307)
at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:301)
at java.lang.ClassLoader.loadClass(ClassLoader.java:248)
Could not find the main class: TestWebCam. Program will exit.
This means the class loader couldn't load the class due to exception in the constructor , You may need to identify the actual exception first to fix.
ReplyDeleteAdd to the class-path of the project the jar file and the native library folder.
ReplyDeleteTo Run the project as jar file , you can use the JVM parameter:
-Djava.library.path=".....\native\win32-x86" to add the library to the class path of the jar file.
i never understand this clearly.
i try these and add jar to project but i got following error:
com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at TestWebCam.(TestWebCam.java:44)
at TestWebCam.main(TestWebCam.java:178)
i cant fix this
please help me.
thanks for reading this.
This means the library is not loaded , if you run using command line you need to add the -D option , if you are running it from inside IDE , go to run options/configurations and add this -D option to Runtime , Java options/arguments.
ReplyDeleteReferring to the problem tuhin is facing, maybe I know how to add it in Eclipse IDE?
ReplyDeleteIt is easily in Eclipse:
ReplyDeleteFrom Eclipse Run --> Open Run Dialog (or Run configuration) --> Arguments tab --> VM Arguments and place -D.....
Getting following error on running the aforementioned program on Ubuntu.
ReplyDeleteIs this some webcam driver issues ? Please help.
Device ID 0: /dev/video0
Description 0: /dev/video0
*** glibc detected *** /usr/lib/jvm/java-6-openjdk/bin/java: free(): invalid pointer: 0xb4d2dcb0 ***
======= Backtrace: =========
/lib/libc.so.6(+0x6c501)[0x224501]
/lib/libc.so.6(+0x6dd70)[0x225d70]
/lib/libc.so.6(cfree+0x6d)[0x228e5d]
/usr/lib/jvm/java-6-openjdk/jre/lib/i386/client/libjvm.so(+0x30885c)[0x12a185c]
/usr/lib/jvm/java-6-openjdk/jre/lib/i386/client/libjvm.so(+0x21a2ec)[0x11b32ec]
/home/local/PERSISTENT/surbhi_gupta/Downloads/lti-civil/native/linux-x86/libcivil.so(_ZN7JNIEnv_18ReleaseStringCharsEP8_jstringPKt+0x1f)[0x409a5f]
/home/local/PERSISTENT/surbhi_gupta/Downloads/lti-civil/native/linux-x86/libcivil.so(Java_com_lti_civil_impl_jni_NativeCaptureSystem_openCaptureDeviceStream+0x12e)[0x408c74]
[0xb572b0dd]
[0xb5724463]
[0xb5723e21]
[0xb5721366]
/usr/lib/jvm/java-6-openjdk/jre/lib/i386/client/libjvm.so(+0x209932)[0x11a2932]
/usr/lib/jvm/java-6-openjdk/jre/lib/i386/client/libjvm.so(+0x30a509)[0x12a3509]
/usr/lib/jvm/java-6-openjdk/jre/lib/i386/client/libjvm.so(+0x20886f)[0x11a186f]
/usr/lib/jvm/java-6-openjdk/jre/lib/i386/client/libjvm.so(+0x213d0c)[0x11acd0c]
/usr/lib/jvm/java-6-openjdk/jre/lib/i386/client/libjvm.so(+0x222d6d)[0x11bbd6d]
/usr/lib/jvm/java-6-openjdk/bin/java(JavaMain+0xd45)[0x804aef5]
/lib/libpthread.so.0(+0x5cc9)[0x1a3cc9]
/lib/libc.so.6(clone+0x5e)[0x2886ae]
======= Memory map: ========
00110000-00132000 r-xp 00000000 08:07 27350 /usr/lib/jvm/java-6-openjdk/jre/lib/i386/libjava.so
00132000-00133000 r--p 00021000 08:07 27350 /usr/lib/jvm/java-6-openjdk/jre/lib/i386/libjava.so
....
....
....
opened v4l2 device
Found UVC Camera (046d:0994) card with uvcvideo v4l2 driver
discover_inputs()
Found sources: 1
0 - Camera 1 (2)
unknown or unsupported format: 1196444237
For me it seems driver issue as the service already discovered but the format is not supported , can you check the supported formats?
ReplyDeleteThanks for the reply.
ReplyDeleteThe program worked for me on windows. Can I capture the webcam images through command line without the swing GUI. I tried but its asking for Quick time. Can you please suggest some alternative ?
Hi Surbahi,can you please guide me How the Image capyure worked in case of you. I also followed the same steps described in blog.but gettinf exception.code is able to detect device
DeleteDSCaptureException::FailWithException: hr=0x800705aa.
com.lti.civil.CaptureException: pMediaControl->Run() failed: 0
at com.lti.civil.impl.jni.NativeCaptureStream.nativeStart(Native Method)
at com.lti.civil.impl.jni.NativeCaptureStream.start(NativeCaptureStream.java:52)
at com.ample.TestWebCam$1.actionPerformed(TestWebCam.java:74)
help will be appricated.
my mail Id is amar_it05@yahoo.com
plz provide me steps I need it urgently
Yea , you can if you refer to other open source projects you can find a way :
ReplyDeletehttp://osama-oransa.blogspot.com/2011/01/hidden-parent-eye.html
http://osama-oransa.blogspot.com/2010/12/java-home-monitor-application.html
Simply remove the JFrame :) or setVisible(false) for it.
thanks for posting this code but i have a question:
ReplyDeletewhat do u mean by: implements CaptureObserver ? the class TestWebCam is abstract?
can u post a full code plz
plz help, i have been searching for days of ways to capture webcam image from java code
When you make it implement this interface you must add these 2 methods to the class: public void onNewImage(CaptureStream stream, Image image) & public void onError(CaptureStream stream, CaptureException ce)...
ReplyDeleteFor a working example plz have a look on the other open source projects in my blog: http://osama-oransa.blogspot.com/2010/12/java-home-monitor-application.html
hi.. I'am new with JMF.. i need to capture image using a laptop webcam. i used JMF it running but it cannot save image i capture which i need most. can you help me?? hopeyou can reply.. god bless
ReplyDeleteThis post is talking about FJM not JMF , you can follow the steps easily or use Interactive4J open source code to do this.
ReplyDeletepublic class SwingCapture extends Panel implements ActionListener
ReplyDelete{
public static Player player = null;
public CaptureDeviceInfo di = null;
public MediaLocator ml = null;
public JButton capture = null;
public Buffer buf = null;
public Image img = null;
public VideoFormat vf = null;
public BufferToImage btoi = null;
public ImagePanel imgpanel = null;
public SwingCapture()
{
setLayout(new BorderLayout());
setSize(320,550);
imgpanel = new ImagePanel();
capture = new JButton("Capture");
capture.addActionListener(this);
String str1 = "vfw:Logitech USB Video Camera:0";
String str2 = "vfw:Microsoft WDM Image Capture (Win32):0";
di = CaptureDeviceManager.getDevice(str2);
ml = di.getLocator();
try
{
player = Manager.createRealizedPlayer(ml);
player.start();
Component comp;
if ((comp = player.getVisualComponent()) != null)
{
add(comp,BorderLayout.NORTH);
}
add(capture,BorderLayout.CENTER);
add(imgpanel,BorderLayout.SOUTH);
}
catch (Exception e)
{
e.printStackTrace();
}
}
public static void main(String[] args)
{
Frame f = new Frame("ImageCapture");
SwingCapture cf = new SwingCapture();
f.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
playerclose();
System.exit(0);}});
f.add("Center",cf);
f.pack();
f.setSize(new Dimension(320,550));
f.setVisible(true);
}
public static void playerclose()
{
player.close();
player.deallocate();
}
public void actionPerformed(ActionEvent e)
{
JComponent c = (JComponent) e.getSource();
if (c == capture)
{
// Grab a frame
FrameGrabbingControl fgc = (FrameGrabbingControl)
player.getControl("javax.media.control.FrameGrabbingControl");
buf = fgc.grabFrame();
// Convert it to an image
btoi = new BufferToImage((VideoFormat)buf.getFormat());
img = btoi.createImage(buf);
// show the image
imgpanel.setImage(img);
// save image
saveJPG(img,"c:\\test.jpg");
}
}
class ImagePanel extends Panel
{
public Image myimg = null;
public ImagePanel()
{
setLayout(null);
setSize(320,240);
}
public void setImage(Image img)
{
this.myimg = img;
repaint();
}
public void paint(Graphics g)
{
if (myimg != null)
{
g.drawImage(myimg, 0, 0, this);
}
}
}
public static void saveJPG(Image img, String s)
{
BufferedImage bi = new BufferedImage(img.getWidth(null), img.getHeight(null), BufferedImage.TYPE_INT_RGB);
Graphics2D g2 = bi.createGraphics();
g2.drawImage(img, null, null);
FileOutputStream out = null;
try
{
out = new FileOutputStream(s);
}
catch (java.io.FileNotFoundException io)
{
System.out.println("File Not Found");
}
JPEGImageEncoder encoder = JPEGCodec.createJPEGEncoder(out);
JPEGEncodeParam param = encoder.getDefaultJPEGEncodeParam(bi);
param.setQuality(0.5f,false);
encoder.setJPEGEncodeParam(param);
try
{
encoder.encode(bi);
out.close();
}
catch (java.io.IOException io)
{
System.out.println("IOException");
}
}
}
can you help me about this code the erros is
Exception in thread "main" java.lang.NullPointerException
at SwingCapture.(SwingCapture.java:39)
at SwingCapture.main(SwingCapture.java:65)
Java Result: 1
This comment has been removed by the author.
ReplyDeleteI am not sure where is the exception line number as you didn't number the post, so most probably you are missing to add the native library to the Java class-path, so try to add this as well..
ReplyDeleteString str2 = "vfw:Microsoft WDM Image Capture (Win32):0";
MR.OSAMA ORASA is it possible that you can program in j2me about browsing mobile inbox message??? if it is possible can you give me a runnable code for it.. thank you very much in advance :))
ReplyDeleteYou can't do this in J2ME but you can use Android for that.
ReplyDeletemr. osama can you hepl me. I'am doing work with computer vision my platform is J2SE in netbeans with JDK 1.6 with JMF. it possible for that?? my project is capture image and save it using Java.
ReplyDeleteIf you follow the post steps you can do this easily.
ReplyDeletegood day, we tried following yours steps like downloading and adding library and a classpath, we seem have a problem configuring the class path since we are using netbeans 7.0 and using jdk 6.0..should we use a higher jdk.. by the way,when we create the program with the codes above, there are so many errors..we can't solve this problem can you help us? specialy on creating class path.. we are very new to this..thank you so much in advance :)
ReplyDeleteit should work fine in netbeans 7.0 and jdk 6.0, do you get compilation errors ? if yes then you didn't add all the jars to class path correctly, if you mean runtime error then you need to load the native lib during running it by using -D
ReplyDeletegood day mr. Osama.i used JMF in java what is the problem about this line: Player player = Manager.createRealizedPlayer(deviceInfo.getLocator()); here the error says:
ReplyDeleteException in thread "main" java.lang.NullPointerException
at Shot.FrameGrab.main(FrameGrab.java:19)
i hope you can reply..
Add System.out.println(""+deviceInfo.getLocator());
ReplyDeleteJust above this line and see if null pointer occur ..
The device info should be initialized by something like (before that line)
String value= "vfw:Microsoft WDM Image Capture (Win32):0";
DeviceInfo deviceInfo = CaptureDeviceManager.getDevice(value);
hey Osama,
ReplyDeleteThanks for the url of ITI-CIVIL in ur blog ....it helped me pretty much..in solving my problem of displaying live video & then capturing the image Thanq..
with regards,
Amala
mr. osama can you hepl me. i dont know about cannot find symbol class capturestream.. iam newbie in java.. thx..
ReplyDeleteAdd the jars to the class path as mentioned above and the compilation error will be resolved.
ReplyDeletemr osama can u help me, im confuse for adding import class requirement for this project.. there's such error like cannot find symbol class ByteArrayInputStream and others.. sory, iam newbie in java..
ReplyDeleteThis means you are not configuring the Java JDK itself correctly, this class should be imported like :
ReplyDeleteimport java.io.ByteArrayInputStream;
Plz check your classpath and add the needed jars and JDK, you can google on how to do this on the IDE you are using.
Hello Sir
ReplyDeleteI am used your code in netbeans
how can used jar file & liabrary file pls tell me
In NetBeans it is easily to add jars to classpath and in run add the JVM param using -D as specified in the steps.
ReplyDeleteHello Sir Can You Pls Send
ReplyDeletelti-civil.zip file on my mail id because some proble of dowloading of your link
pls sir
my email id is : nikunjrathod77@gmail.com
Library sent to your email.
ReplyDeletehello sir i have this error, im using netbeans..
ReplyDeleteException in thread "AWT-EventQueue-0" java.lang.NullPointerException
at aa.TestWebCam$1.actionPerformed(TestWebCam.java:60)
at javax.swing.AbstractButton.fireActionPerformed(AbstractButton.java:1995)
at javax.swing.AbstractButton$Handler.actionPerformed(AbstractButton.java:2318)
at javax.swing.DefaultButtonModel.fireActionPerformed(DefaultButtonModel.java:387)
at javax.swing.DefaultButtonModel.setPressed(DefaultButtonModel.java:242)
at javax.swing.plaf.basic.BasicButtonListener.mouseReleased(BasicButtonListener.java:236)
at java.awt.Component.processMouseEvent(Component.java:6267)
This means you have no USB connected to the machine.
ReplyDeletemr osama, i can running the program.. can I see the image area that will captured by the webcam? and can i capture continuously from webcam within the specified time? thanks in advance
ReplyDeleteYea, you can of-course , you check another open source project Interactive4J in this blog contain example of how to make video/images from web cam in easy way , you can take the source code and use it directly.
ReplyDeleteHello Sir
ReplyDeletehttp://sourceforge.net/projects/lti-civil/files/
this link is not working properly so pls send me
LTI-CIVIL.zip file
my email id is
nikunjrathod77@gmail.com
It is working fine with me , please try to connect using different internet connection.
ReplyDeleteSend failed due to security error as the zip contain some executable files, so please try to download it.
thank you sir
ReplyDeletei can download .zip file
sir i am very new in java so how can set the
jar file and native liabrary
i was using jdk1.6 and the java class path is
c:/j2sdk1.4.2/bin
so pls tell me how can set .jar file and native file i was using window
There is only 1 advice try to use Google to do what you want :) , how did you get my blog ? by search , so you need to do the same for each issue you face , here is a link for this : http://javahowto.blogspot.com/2006/06/set-classpath-in-eclipse-and-netbeans.html
ReplyDeleteHello OSAMA, thx for this nice POST....i am trying ur Code but i get an exception,
ReplyDeleteu allready answer that we hav to add an argument to eclipse, but can u explain wich argument i have to write in VM argument window? Thx.....
Exception is:
com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at TestWebCam.(TestWebCam.java:48)
at TestWebCam.main(TestWebCam.java:163)
Add this argument:
ReplyDelete-Djava.library.path=".....\native\win32-x86"
..... = parent folders of this Civil library.
how to add this argument?
DeleteJVM parameters or run parameters.
Deletei want to access this applet in jsp page How can I?
ReplyDeleteThere is another post describing that for using web cam in JSP file.
DeleteUsing the applet in JSP page is the same as using it in any normal html.
ReplyDeleteBut You can't use this in applet because of the Native Library.
why is this?
ReplyDeleteerror: package com.sun.image.codec.jpeg does not exist
import com.sun.image.codec.jpeg.JPEGCodec;
isnt this package part of the API??
im through JDK 7.
No , it is not , you can replace it with your own code. or use old JDK.
Deleteit might be removed as it is Sun file and could be removed at any JDK release.
DeleteFrom the Java Docs 1.5 you can see: "This class is a factory for implementations of the JPEG Image Decoder/Encoder.
ReplyDeleteNote that the classes in the com.sun.image.codec.jpeg package are not part of the core Java APIs. They are a part of Sun's JDK and JRE distributions. Although other licensees may choose to distribute these classes, developers cannot depend on their availability in non-Sun implementations. We expect that equivalent functionality will eventually be available in a core API or standard extension."
thanks for the reply..hmm..i got it... sooo..what I shal do?
ReplyDeleteany alternatives? pls.
Hi,
ReplyDeleteI have tried to create all classes in "com.sun.image.codec.jpeg" and refer to those classes without refering to the particular package.
Will it work out??
while im trying to implement this strategy.
I have got strucked at following classes,
JPEGEncodeParam and JPEGQTable.
You may include the JAR file from the JRE or get them from Sun JRE as a source code and include them in your project if this is includes a small set of classes, or you can search for any other option available on the internet to provide the same function of encoding.
ReplyDeleteok..thank you for your time. ill try ma best. regards.
ReplyDeletehello i m beginner java user how we set class path in netbeans
ReplyDeleteI advice you to search on every thing using Google and you will find the answer...
ReplyDeletehttp://javahowto.blogspot.com/2006/06/set-classpath-in-eclipse-and-netbeans.html
can i use this library in applet?
ReplyDeleteNo , you can't >>> you can use web start instead...
ReplyDeleteHi Osama,
DeleteCould you explain me please how to load this application as a java web start application?
Thanks in advance.
Use this tutorial :
Deletehttp://www.mkyong.com/java/java-web-start-jnlp-tutorial-unofficial-guide/
hello sir i have this error, im using netbeans..
ReplyDeleteException in thread "AWT-EventQueue-0" java.lang.NullPointerException
at aa.TestWebCam$1.actionPerformed(TestWebCam.java:60)
at javax.swing.AbstractButton.fireActionPerformed(AbstractButton.java:1995)
at javax.swing.AbstractButton$Handler.actionPerformed(AbstractButton.java:2318)
at javax.swing.DefaultButtonModel.fireActionPerformed(DefaultButtonModel.java:387)
at javax.swing.DefaultButtonModel.setPressed(DefaultButtonModel.java:242)
at javax.swing.plaf.basic.BasicButtonListener.mouseReleased(BasicButtonListener.java:236)
at java.awt.Component.processMouseEvent(Component.java:6267)
August 4, 2011 8:00 AM
whereas my webcam is connected to machine
please suggest some advice
This means USB camera is not connected or not recognized , can you try it with another program that uses it.
ReplyDeletehi sir, when i click start button i am able to see video streaming.. could you help me please, what is the problem
ReplyDeleteYou can click on the image to change it , or create a thread that do the same to refresh the image every x time , may be 1 second according to the smooth appearance you want to have... the less the time the more the smooth you get.
ReplyDeleteHi sir gud mor i followed ur steps even i dnt ve any prob in compiling but when i click the start button my web cam is not opening can u provide me the details !!!
ReplyDeletei am facing dis prob in Eclipse tool
Any exception is thrown ? can you test your web cam using another application as well ? is the java class path is set correctly and dynamic library ? can you try running the jar from the command line ?
ReplyDeleteMy web cam is running properly pls can u send me the steps to run through command prompt...
ReplyDeleteThanks for ur previous reply!!
Use java -jar your_jar_name -Djava.library.path=".....\native\win32-x86"
ReplyDelete-D will load the native library...
HI SIR,Can u say how to Save the image in a gray format , i followed all ur steps every thing s working fine but the problem arises when saving the image it s being saved only in coloured format even though i apply gray scale conversion as u specified!!!looking for ur reply!!
ReplyDeleteAfter converting you need to save the image : so save myImage here :
ReplyDelete//Convert myImage to gray scale
BufferedImage imageGray = new BufferedImage(myImage.getWidth(), myImage.getHeight(),
BufferedImage.TYPE_BYTE_GRAY);
Graphics g = imageGray.getGraphics();
g.drawImage(myImage, 0, 0, null);
***** Save the image here ******
g.dispose();
shot.setIcon(new ImageIcon(imageGray));
This solution run over windows 7 of 64 bits
ReplyDeletehow to run it am not able to get it plzz help me.explain in detail plz
ReplyDeleteSorry , what was your issue , explain so i can help.
ReplyDeletePlease help me .How to display live video from webcam on my Frame. .and from that live video i want to take a picture.
ReplyDeleteFollow the steps and create a thread to capture the image in a rate (the fast the better may be 1 image per seconds or 12 per seconds)..display the images will give you the feeling of the video and you can store the image you want.
ReplyDeleteHi sir,Please help me.I want to display a live video from my webcam on my jsp page using jmf (As you said that we cannot use fmj because of native library).
ReplyDeleteI think the only way is to use plug-in for the browser , and i can see many sites using Flash Player for that as it can capture from webcam.
ReplyDeleteHi sir,i get following error during run time..please help me to overcome it..please..
ReplyDeleteException in thread "main" com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: /home/liveuser/lti-civil/native/linux-x86/libcivil.so: libstdc++.so.5: cannot open shared object file: No such file or directory
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at Cam.main(Cam.java:26)
Caused by: java.lang.UnsatisfiedLinkError: /home/liveuser/lti-civil/native/linux-x86/libcivil.so: libstdc++.so.5: cannot open shared object file: No such file or directory
at java.lang.ClassLoader$NativeLibrary.load(Native Method)
at java.lang.ClassLoader.loadLibrary0(ClassLoader.java:1807)
at java.lang.ClassLoader.loadLibrary(ClassLoader.java:1732)
at java.lang.Runtime.loadLibrary0(Runtime.java:823)
at java.lang.System.loadLibrary(System.java:1028)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:21)
... 1 more
I can see the following exception : java.lang.UnsatisfiedLinkError means the native library can't be linked , so check the path : /home/liveuser/lti-civil/native/linux-x86/libcivil.so
DeleteCheck the privileges of this user to access it , and check the Linux/OS version you are using..
In Fedora14 linux i run a program that captures image from usb Webcam gives error like
ReplyDelete[liveuser@localhost bin]$ /home/liveuser/lti-civil/bin/java -classpath we.jar -Djava.library.path="native/linux-x86" com.lti.civil.test.Initial
Device ID 0: /dev/video0
Description 0: /dev/video0
opened v4l2 device
Found USB2.0 PC CAMERA card with uvcvideo v4l2 driver
discover_inputs()
Found sources: 1
0 - Camera 1 (2)
unknown or unsupported format: 1448695129
Exception in thread "main" com.lti.civil.CaptureException: unknown or unsupported format: 1448695129
at com.lti.civil.impl.jni.NativeCaptureSystem.openCaptureDeviceStream(Native Method)
at com.lti.civil.test.Initial.main(initial.java:46)
Sir,kindly help..why this is happening and how can i resove it??
The camera is detected but it can't open the device to capture image , are you able to use it from other programs with no issues ?
Deleteyes sir. i can capture image by cheese program in fedora 14 but through java code using lti-civil..the upper mentioned runtime error is occuring..
ReplyDeleteSir please help,what should i do?
how to make jar file tht run on all computers
ReplyDeleteCreate 1 jar file and create .bat or .sh files for each system that refer to the native libraries.
DeleteI was Trying to run above program in NETBEANS 7 but i got error please help me..
ReplyDeleteException in thread "main" java.lang.RuntimeException: Uncompilable source code - incompatible types
required: CaptureSystemFactory
found: com.lti.civil.impl.jni.NativeCaptureSystemFactory
at DefaultCaptureSystemFactorySingleton.instance(DefaultCaptureSystemFactorySingleton.java:24)
at TestWebCam.(TestWebCam.java:25)
at TestWebCam.main(TestWebCam.java:87)
This seems conflicts in your class-path check the jars (remove unnecessary) and retry...
DeleteHi,
ReplyDeleteI am using java1.6 for running the prog given by u....i am getting 4 warning in cmd.........
com.sun.image.codec.jpeg.* is Sun proprietary API nad may be removed in future release
Sir can u plz suggest me the solution for that.......nd also tried to install jdk1.5 but my processor is not compatible with it.......... plz rply as soon as possible.....i need it!!!
thanks
Ignore these warnings or use suppress warnings.
DeleteThanks,
Osama
I have the following exceptions and it doest not show anything an clicking START button nd i am using jdk1.6:
ReplyDeleteException :
com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java
.library.path
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem
(NativeCaptureSystemFactory.java:24)
at TestWebCam.(TestWebCam.java:45)
at TestWebCam.main(TestWebCam.java:141)
Caused by: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at java.lang.ClassLoader.loadLibrary(Unknown Source)
at java.lang.Runtime.loadLibrary0(Unknown Source)
at java.lang.System.loadLibrary(Unknown Source)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem
(NativeCaptureSystemFactory.java:21)
... 2 more
Plz rply fast...........
Please specify the native library path correctly refer to point#2.
DeleteSir i have given
ReplyDeleteclasspath
variable value = D:\new webcam\lti-civil-20070920-1721\native\win32-x86;D:\new webcam\lti-civil-20070920-1721\lti-civil-no_s_w_t.jar;
is anything extra to be done???
no, you should configure the native library as well , 1st parameter in your class-path is native library ... so it should be passed as parameter to the JVM: -D........
ReplyDeleteThis is a JVM parameter and need to be set from Run properties in Netbeans or eclipse or from the command line if you run your project from the command line.
Thanks a lot sir,
ReplyDeleteThe prog run smoothly in netbeans but i still could run it in command line............and can u give some idea of video capturing also to be embedded with this program only!!!!!!!!!!
I shall be highly thankful to you............
For video capturing , simply create a thread to capture the images in speed x images per seconds and put it in the same place it will looks like a video exactly.
DeleteThe other option , you can download my Interactive4J open source and get the needed code to do the same.
Sir,
ReplyDeleteCan u plz tell me exactly where to use thread i.e for which func in prog u have given above for vedio..actually i have tried alot but it is not working properly!!!!!!!!!
i shall be thankful to u.........
and thanks for info u have given me!!!!!!!!
Please check my open source code , it do all what you need.
DeleteThanks..
i am using netbeans ide and i added a lib directory to libraries consisting of lti-civil-no_s_w_t.jar and swt.jar and i am using w7 32 bit so i added the dl folders accoring to it on the lib
ReplyDeletebut when i try to run the file
om.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at googlecv.TestWebCam.(TestWebCam.java:54)
at googlecv.TestWebCam.main(TestWebCam.java:160)
Caused by: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at java.lang.ClassLoader.loadLibrary(ClassLoader.java:1860)
at java.lang.Runtime.loadLibrary0(Runtime.java:845)
at java.lang.System.loadLibrary(System.java:1084)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:21)
... 2 more
this error s showing can u please tell me how to resoulve this
hi i am using netbeans to run the above code
ReplyDeletei am using windows7 os 32 bit
i added a lib folder containing lti-civil-no_s_w_t.jar and swt.jar and the .dll files needed for windows 32 bit
but when i try to run the above code i am getting this error . can u please tell me how to fix it
om.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at googlecv.TestWebCam.(TestWebCam.java:54)
at googlecv.TestWebCam.main(TestWebCam.java:160)
Caused by: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at java.lang.ClassLoader.loadLibrary(ClassLoader.java:1860)
at java.lang.Runtime.loadLibrary0(Runtime.java:845)
at java.lang.System.loadLibrary(System.java:1084)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:21)
... 2 more
Please add the jvm parameter -D ...... with the correct library.
DeleteSir, thanks for previous info nd i am able to run vedio and image capturing smoothly ...........
ReplyDeletecan sir plz help me out in making face detection and face comparison application.....actully i have done face detection but could save the image and compare with other saved image...............
thanks in advance.......
plz help me out!!!!!!!!!!!!
This need statisitcal analysis, so you need to dig more on statistical analysis.
DeleteHi thanks for the code help. I want to capture image automatically with the webcam. The user should not click to capture the image. I had used JTwain.jar and Asprise.dll but its a trial version. Can u plz help i am in very much need of it.
ReplyDeleteThanks
You can check the code in Interactive4J for that or Home monitor application..
DeleteI want to capture image from my web application through applet,can i use this library in my applet code.
ReplyDeleteThanks in advance.......
Please help me out
Unfortunally no .. search for another way..
DeleteThanks for your valuable post,
ReplyDeleteI got this application executed, but I found that it doesn't show the video preview of the webcam so that we can take the exact snapshot of the video(image).
Could you please help to resolve this issue.
Thanks & Regards,
Rasheed
You can show the video by using a thread to capture the images in the speed you need e.g. 14 frame per second, you may use this in my open source project Interactive4J.
DeleteThank you so much for your reply.
DeleteI tried your suggestion to display the video preview of the webcam, but I couldn't get the exact location to put the code, I request you to provide the code to get the webcam video.
Thank you very much.
Regards,
Rasheed
send me your email.
DeleteHi Rasheed,
DeleteHere is a new post talking about how to capture video in your application..
http://osama-oransa.blogspot.com/2012/04/webcam-video-capture-in-java.html
Thanks,
Osama
I've opened the url above, but I couldn't get the exact location to put the code, I request you to provide the code to get the webcam video.
ReplyDeleteThank you very much. tory
Can you download interactive4j , you will find all the details ... the post is explaining it.
Deletethanks, now I can capture the picture with the camera privew. But can you help me to set the image size? tahnks
Deletedude thx 4 sharing! but i've got little problem here. it's on an exception when i press start button
ReplyDeleteException in thread "AWT-EventQueue-0" java.lang.UnsatisfiedLinkError: com.lti.civil.impl.jni.NativeCaptureStream.start()V
at com.lti.civil.impl.jni.NativeCaptureStream.start(Native Method)
at ticketing.WebCam$1.actionPerformed(WebCam.java:56)
at javax.swing.AbstractButton.fireActionPerformed(AbstractButton.java:2018)
at javax.swing.AbstractButton$Handler.actionPerformed(AbstractButton.java:2341)
at javax.swing.DefaultButtonModel.fireActionPerformed(DefaultButtonModel.java:402)
at javax.swing.DefaultButtonModel.setPressed(DefaultButtonModel.java:259)
at javax.swing.plaf.basic.BasicButtonListener.mouseReleased(BasicButtonListener.java:252)
at java.awt.Component.processMouseEvent(Component.java:6504)
at javax.swing.JComponent.processMouseEvent(JComponent.java:3321)
at java.awt.Component.processEvent(Component.java:6269)
at java.awt.Container.processEvent(Container.java:2229)
at java.awt.Component.dispatchEventImpl(Component.java:4860)
at java.awt.Container.dispatchEventImpl(Container.java:2287)
at java.awt.Component.dispatchEvent(Component.java:4686)
at java.awt.LightweightDispatcher.retargetMouseEvent(Container.java:4832)
at java.awt.LightweightDispatcher.processMouseEvent(Container.java:4492)
at java.awt.LightweightDispatcher.dispatchEvent(Container.java:4422)
at java.awt.Container.dispatchEventImpl(Container.java:2273)
at java.awt.Window.dispatchEventImpl(Window.java:2713)
at java.awt.Component.dispatchEvent(Component.java:4686)
at java.awt.EventQueue.dispatchEventImpl(EventQueue.java:707)
at java.awt.EventQueue.access$000(EventQueue.java:101)
at java.awt.EventQueue$3.run(EventQueue.java:666)
at java.awt.EventQueue$3.run(EventQueue.java:664)
at java.security.AccessController.doPrivileged(Native Method)
at java.security.ProtectionDomain$1.doIntersectionPrivilege(ProtectionDomain.java:76)
at java.security.ProtectionDomain$1.doIntersectionPrivilege(ProtectionDomain.java:87)
at java.awt.EventQueue$4.run(EventQueue.java:680)
at java.awt.EventQueue$4.run(EventQueue.java:678)
at java.security.AccessController.doPrivileged(Native Method)
at java.security.ProtectionDomain$1.doIntersectionPrivilege(ProtectionDomain.java:76)
at java.awt.EventQueue.dispatchEvent(EventQueue.java:677)
at java.awt.EventDispatchThread.pumpOneEventForFilters(EventDispatchThread.java:211)
at java.awt.EventDispatchThread.pumpEventsForFilter(EventDispatchThread.java:128)
at java.awt.EventDispatchThread.pumpEventsForHierarchy(EventDispatchThread.java:117)
at java.awt.EventDispatchThread.pumpEvents(EventDispatchThread.java:113)
at java.awt.EventDispatchThread.pumpEvents(EventDispatchThread.java:105)
at java.awt.EventDispatchThread.run(EventDispatchThread.java:90)
The native library is not linked correctly, please refer to the post again in the -D parameter .. or see previous replies for the same issue.
DeleteSir I want to use webcame code in jsp can u help me......?
ReplyDeleteHello,
ReplyDeleteI did all steps that you described at the beginning, first I got:
com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
After setting PATH=C:\Users\mike\Desktop\lti-civil-20070920-1721\native\win32-x86
I get the following error:
com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: C:\Users\mike\Desktop\lti-civil-20070920-1721\native\win32-x86\civil.dll: Can't load IA 32-bit .dll on a AMD 64-bit platform
Maybe you can help me out?
Thanks!
As in the exception , you are linking 32 bit library in 64 bit system, so either you use a specified library for 64 (if they released one for it) or you need to work around it by run it as 32 (i don't know how) or install a virtual machine for 32 bit operating system.
DeleteSir, firstly i salute you for your valuable post and more knowledge in java programming, today i get yours "lti-civil-20070920-1721" file and i also run file in netbeans 6.8 and set all class path but still it gives error :
ReplyDeleteException in thread "main" com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24) at com.lti.civil.test.CaptureSystemTest.main(CaptureSystemTest.java:32)
Caused by: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at java.lang.ClassLoader.loadLibrary(ClassLoader.java:1734)
at java.lang.Runtime.loadLibrary0(Runtime.java:823)
at java.lang.System.loadLibrary(System.java:1028)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:21)
... 1 more
Java Result: 1
please help me i use it newly and i need it.
Sir, firstly i saluate you for your valuable post and best knowledge in java programming, today i get your project and also run in netbeans 6.8 and also set all classpath but it still gives error :
ReplyDelete"Exception in thread "main" com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at com.lti.civil.test.CaptureSystemTest.main(CaptureSystemTest.java:32)
Caused by: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at java.lang.ClassLoader.loadLibrary(ClassLoader.java:1734)
at java.lang.Runtime.loadLibrary0(Runtime.java:823)
at java.lang.System.loadLibrary(System.java:1028)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:21)
... 1 more
Java Result: 1"
Sir please help me i need it
You need to link the native library using the java parameter -D......location of the library
DeletePlease refer to the post or other comments....
can u mail me a simple code for capturing an image and saving it in a folder just when i run the program and using JMF and java inbuilt classes only??
ReplyDeletei nee it for my college project and cant find a solution since last 6 days...please help me out
Any of my open source project contain the code ready: home monitor or interactive4j is better.
ReplyDeletesir! is there any difference instead of using a webcam i will use a digital camera? if there is, do you have a code for that? thanks in advance..
ReplyDeleteThis should work with usb connected camera.
Deletesir! i have a question:
ReplyDeletehow can i show the preview of the image that i want to capture before capturing it? and on what part of the program i should modify in order to solve this problem!..
i'm a newbie in java.. i am developing a java app that includes capturing images using a webcam or digital camera..
hoping for your quick response.. i need it badly..
please refer to Interactive4J open source project to do this.
DeleteHi, I am using netbeans 7 and jdk1.7.0_04. I have tried the code given in "Capture photo / image from Web Cam / USB Camera using Java". But I am getting error at method info.getDeviceID() and also it is giving error at class defination, saying make class TestWebCam abstract. Please help me.
ReplyDeleteSearch for similar exception in other comments and the reply to these exceptions, otherwise post the exception.
DeleteHi Thanks for your valuable post.This standalone application works fine.But when i integrate same code from applet to jsp i am getting errors like this
ReplyDeleteException: java.lang.NoClassDefFoundError: com/lti/civil/CaptureObserver
java.lang.NoClassDefFoundError: com/lti/civil/CaptureObserver
at java.lang.ClassLoader.defineClass1(Native Method)
at java.lang.ClassLoader.defineClassCond(Unknown Source)
at java.lang.ClassLoader.defineClass(Unknown Source)
at java.security.SecureClassLoader.defineClass(Unknown Source)
at sun.plugin2.applet.Applet2ClassLoader.findClass(Unknown Source)
at sun.plugin2.applet.Plugin2ClassLoader.loadClass0(Unknown Source)
at sun.plugin2.applet.Plugin2ClassLoader.loadClass(Unknown Source)
at sun.plugin2.applet.Plugin2ClassLoader.loadClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
at sun.plugin2.applet.Plugin2ClassLoader.loadCode(Unknown Source)
at sun.plugin2.applet.Plugin2Manager.createApplet(Unknown Source)
at sun.plugin2.applet.Plugin2Manager$AppletExecutionRunnable.run(Unknown Source)
at java.lang.Thread.run(Unknown Source)
plz help me.
There is another post to address how to use web cam in JSP file in m blog : http://osama-oransa.blogspot.co.uk/2012/06/accessing-webcam-within-jsp.html
DeleteFor me its perfectly working. can some one help me to get webcam settings or property panel.
ReplyDeleteFor me its working perfectly. someone can help me out to get webcam setting or property panel by using this library.
ReplyDeletehello, btw i want to make a project that is almost the same as this one, but i hardly understand your explanation above...i am still a newbie on java and this is a fundamental project for me to understand more about it...btw i am using netbeans 7 and i use netbeans UI designer to design the main interface of my application...do you have at least the working source code from your explanation above? thx for replying
ReplyDeleteif u dont understand this post , go to my open source Interactive4j.
Deleteon your link :
ReplyDeletehttp://osama-oransa.blogspot.com/2012/04/webcam-video-capture-in-java.html
you say this :
"You class must implements : CaptureListener , this interface has 1 method inside it :
public void setCaptureFile(File filename);"
what do you mean by this?
i have already made a surface class for that, but do i have to re-define it again in main class?
sir,,
ReplyDeletei have run these codes,but when it capture, it indicate only the black screen.
but there was no error and also show in my programme and it save in correct position in my laptop...
can you tell why it indicate only black screen when i captured photo..
What do you mean by black ? also does it save the correct image or it is also black one ?
Deletesir ,
Deleteit save as black color photo.
remove step 7 that convert it from color to gray scale.
Delete1.com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
ReplyDelete2.Exception in thread "AWT-EventQueue-0" java.lang.NullPointerException
How Can i Overcome This Exception
add jvm parameter -d as specified above..
DeleteI have created this application in netbeans7.1 & jdk1.7.
ReplyDeleteWhen i want to run this application Following Error Occur How can i solve this...
com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at javaapplication4.TestWebCam.(TestWebCam.java:37)
at javaapplication4.TestWebCam.main(TestWebCam.java:149)
Caused by: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at java.lang.ClassLoader.loadLibrary(ClassLoader.java:1860)
at java.lang.Runtime.loadLibrary0(Runtime.java:845)
at java.lang.System.loadLibrary(System.java:1084)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:21)
Plz, Help me..
add jvn parameter -d as specified above.
DeleteiKndly Tell me how can i add the jvn parameters Kindly Help me
DeleteIn the project properties in netbeans you will find run properties where there is an option for JVM parameters.
Deletehello friend, I can´t make it work my "jar" when I run I do not see anything and tried with the command line like this java -jar c: \ CapturaFram.jar -Djava.library.path = "c: \ win32 -x86 "
ReplyDeletebut mark "error in the main, not civil in java library path"
help me please
This means library is not linked corre
Deletectly is your windows 32 bit ? is the library in this path?
Hi Osama Oransa!
ReplyDeleteDo u know how to setting webcam resolution using lti-civil?
I didn't do that before but What kind of settings?
DeleteSome can be developed by yourself as captured frame per second.
I used the following code and the error shown to me is
ReplyDeletecom.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at com.pac.TestWebCam.(TestWebCam.java:62)
at com.pac.TestWebCam.main(TestWebCam.java:203)
Caused by: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at java.lang.ClassLoader.loadLibrary(ClassLoader.java:1860)
at java.lang.Runtime.loadLibrary0(Runtime.java:845)
at java.lang.System.loadLibrary(System.java:1084)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:21)
i have imported the jar and the native folder ...
I am using windows 7 and jdk1.7 and netbeans 7.2 verson
Thanks in advance
I am getting the error
ReplyDeletecom.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
what is the problem
Thanks in advance
Native library is not correctly located/defined.
Deletehello sir!
ReplyDeleteon what particular class can i set the frame rate in order for me to view the exact movement i want to capture.. coz i'm making a project that would capture images.. but the problem is that the preview is in slow motion.. hoping for your immediate response.. thank you in advance..
You can control this by decreasing your loop, post your code so i can assist you.
DeleteYou may use my open source Interactive4J directly to capture video.
is it possible to adjust the frame rate for the preview? i'm using your lti-civil-no_s_w_t jar file.. coz the frame rate is too slow, i want it to be more faster.. tnx.
ReplyDeleteYou can control this by decreasing your loop, post your code so i can assist you.
Deletehow can i change screen resolution
ReplyDeleteException in thread "AWT-EventQueue-0" java.lang.UnsatisfiedLinkError: com.lti.civil.impl.jni.NativeCaptureStream.start()V
ReplyDeleteat com.lti.civil.impl.jni.NativeCaptureStream.start(Native Method)
How can i fix this problem, please?
Sir Can u plz help me.
ReplyDeleteEverything is working except when i click on START button the WEBCAM is initialized and started but I can't see the actual streaming. After clicking on SHOT the image is displayed properly, but initially after START nothing is visible in the frame.
Can u plz help.
I want the (Camera)Live streaming to be visible after clicking on Start. Rest all is fine.
Plz can u modify or help me with ur same code.
Dear Osama Oransa,
ReplyDeleteI am developing web application for capturing images from webcam. I have some issues with JMF. it doesn't recognize usb webcam. so i am planning to switch over to lti.civil. So i tried the code which you have given in the same post. its working fine. then i converted the same in to applet its not working. my plan is to embed the applet in jsp. its giving noclassdeffcoundErro com/lti/civil/captureException. I signed applet using keytool. Is it the problem with accessing native using tomcat5.5 please help me solving the problem . Thank you
Please look at the other post describing how to do that for JSP files.
ReplyDeleteIm new to this. i used this code nd im getting following error
ReplyDeleteava.lang.ExceptionInInitializerError
Caused by: java.lang.RuntimeException: Uncompilable source code - onNewImage(com.lti.civil.CaptureStream,com.lti.civil.Image) is already defined in webcam.TestWebCam
at webcam.TestWebCam.(TestWebCam.java:107)
Exception in thread "main" Java Result: 1
thanks!!
ReplyDeletehey...I am working on a project where i need to use webcam.they above code is working perfectly but can i see the Pre-stream of webcam what i am going to captr on cilckevent of button. Please Reply soon.
ReplyDeleteCan you please check interactive4J project , this is done in the demo ...
DeleteThanks..
Its seems this post is too old!!!
ReplyDeletebut am getting this error,iam using netbeans 7.3
com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at testwebcam.TestWebCam.(TestWebCam.java:51)
at testwebcam.TestWebCam.main(TestWebCam.java:154)
Caused by: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at java.lang.ClassLoader.loadLibrary(ClassLoader.java:1860)
at java.lang.Runtime.loadLibrary0(Runtime.java:845)
at java.lang.System.loadLibrary(System.java:1084)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:21)
... 2 more
If you looked at previous comments you will find the answer.
Delete-You didn't put the native library in Java classpath so it can load it , please use -D as described above:
-Djava.library.path=".....\native\win32-x86" to add the library to the class path of the jar file.
You can add it in netbeans in the run JVM argument
I have added the jar file in my project .Also copied the native lib folder and pasted into my project application folder .
ReplyDeleteDo i need to set class path explicity.
I run all other projects with the class path already set
Please follow the steps and add -D option during execution so the native library cab be loaded :)
DeleteHi can this work on windows 8 64 bit?
ReplyDeletehello friends, to not have problems with JPEG library, I leave the following code, which will allow them to create the .jar
ReplyDeleteonly change the method onNewImage
@Override
public void onNewImage(CaptureStream stream, Image image) {
if(!takeShot) return;
takeShot=false;
System.out.println("New Image Captured");
byte[] bytes = null;
try {
if (image == null) {
bytes = null;
return;
}
//File file = new File("fotos/img" +Calendar.getInstance().getTimeInMillis() +".jpg");
file=new File("fotos/imgpaciente.jpg");
final BufferedImage bufferedImage = AWTImageConverter.toBufferedImage(image);
ImageIO.write(bufferedImage, "JPG", file);
img.setText("");
img.setIcon(new ImageIcon(bufferedImage));
img.revalidate();
} catch (IOException ex) {
ex.printStackTrace();
}
}
hello friends and thank you osama, to not have problems with JPEG library, I leave the following code, which will allow them to create the .jar
ReplyDeleteonly change the method onNewImage
@Override
public void onNewImage(CaptureStream stream, Image image) {
if(!takeShot) return;
takeShot=false;
System.out.println("New Image Captured");
byte[] bytes = null;
try {
if (image == null) {
bytes = null;
return;
}
//File file = new File("fotos/img" +Calendar.getInstance().getTimeInMillis() +".jpg");
file=new File("fotos/imgpaciente.jpg");
final BufferedImage bufferedImage = AWTImageConverter.toBufferedImage(image);
ImageIO.write(bufferedImage, "JPG", file);
img.setText("");
img.setIcon(new ImageIcon(bufferedImage));
img.revalidate();
} catch (IOException ex) {
ex.printStackTrace();
}
}
Hello sir,
ReplyDeletei try your code i got some error, as shown in below:
com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at TestWebCam.(TestWebCam.java:106)
at TestWebCam.main(TestWebCam.java:166)
Caused by: java.lang.UnsatisfiedLinkError: no civil in java.library.path
at java.lang.ClassLoader.loadLibrary(ClassLoader.java:1886)
at java.lang.Runtime.loadLibrary0(Runtime.java:849)
at java.lang.System.loadLibrary(System.java:1088)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:21)
... 2 more
This is because the native library is not configured correctly, please fix this by using the library location as in your computer...
Deleteex = (com.lti.civil.CaptureException) com.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: /home/ketangspl/Downloads/lti-civil-20070920-1721/native/linux-x86/libcivil.so: libstdc++.so.5: cannot open shared object file: No such file or directory
ReplyDeleteI am facing such problems... Can you please guide me what shall be the problem
The library seems to be missing or you do not have the grants to access it in that location:
Delete/home/ketangspl/Downloads/lti-civil-20070920-1721/native/linux-x86/libcivil.so
Check the path and library file name.
Hi Osama, i have this error and i am using windows8,Netbeans
ReplyDeletecom.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: G:\vinismon\NetbeansSampleProgram\TestWebCam\civil.dll: Can't load IA 32-bit .dll on a AMD 64-bit platform
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:24)
at testwebcam.TestWebCam.(TestWebCam.java:41)
at testwebcam.TestWebCam.main(TestWebCam.java:139)
Caused by: java.lang.UnsatisfiedLinkError: G:\vinismon\NetbeansSampleProgram\TestWebCam\civil.dll: Can't load IA 32-bit .dll on a AMD 64-bit platform
at java.lang.ClassLoader$NativeLibrary.load(Native Method)
at java.lang.ClassLoader.loadLibrary1(ClassLoader.java:1965)
at java.lang.ClassLoader.loadLibrary0(ClassLoader.java:1890)
at java.lang.ClassLoader.loadLibrary(ClassLoader.java:1880)
at java.lang.Runtime.loadLibrary0(Runtime.java:849)
at java.lang.System.loadLibrary(System.java:1088)
at com.lti.civil.impl.jni.NativeCaptureSystemFactory.createCaptureSystem(NativeCaptureSystemFactory.java:21)
... 2 more
Exception in thread "main" java.lang.RuntimeException: Uncompilable source code - Erroneous ctor sym type:
at testwebcam.TestWebCam.(TestWebCam.java:62)
at testwebcam.TestWebCam.main(TestWebCam.java:139)
Picked up _JAVA_OPTIONS: -Xmx512M
Java Result: 1
BUILD SUCCESSFUL (total time: 6 seconds)
You need to use the correct native library for 64 bit not the one for 32 bit.
Deletehello sir i want to capture the face displayed on webcam and save the image to a folder on my computer can you help me
ReplyDeleteFollow the steps and save the file, don't see any issue in what you want.
DeleteHi sir can you clearly explain how to fix the library?
ReplyDeletecom.lti.civil.CaptureException: java.lang.UnsatisfiedLinkError: no civil in java.library.path
Please back to the steps and ensure the native library is in right location and referenced by -D parameter correctly.
DeletePlz explain me step by step, i dont get it
DeleteCan you refer to step 1 and 2 above ...
DeleteDoes the win32 *86 works for a 64 bits?
Deleteif yes, its this part that i dont get :
Add to the class-path of the project the jar file and the native library folder.
To Run the project as jar file , you can use the JVM parameter:
-Djava.library.path=".....\native\win32-x86" to add the library to the class path of the jar file.
I don't think so.
Deleteif i want to use webcam what should i do in this code ???
ReplyDeleteif the devise is recognized, just connect in the correct one you need.
DeleteThe code already loop over existing devices and print them.
I have error at this statement if(!takeShot) return;
ReplyDeleteWhat error ? can you post here ?
DeleteHI, I have executed the code and it runs fine, thanks. But i kindly request that you do direct me to where i could get documentation about this LTI-CIVIL library iv'e searched online but cant seem to get any useful documentation about it.
ReplyDelete