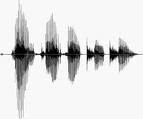
Speech recognition is one of the challenging areas in computer science, a lot of pattern recognition methodology tried to resolve a good way and higher percentage of recognition.
One of the best ways to be use is Hidden Markov Model :
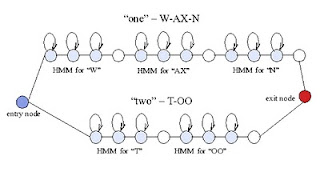
"The process of speech recognition is to find the best possible sequence of words (or units) that will fit the given input speech. It is a search problem, and in the case of HMM-based recognizers, a graph search problem. The graph represents all possible sequences of phonemes in the entire language of the task under consideration. The graph is typically composed of the HMMs of sound units concatenated in a guided manner, as specified by the grammar of the task."
There are a lot of Java Based Speech Recognition Engines, you can find them here:
http://ocvolume.sourceforge.net/links.php.
In this post we will select one of the famous open source Java speech recognition software and identify simple steps to use it.
Sphinx-4
Sphinx-4 is a state-of-the-art speech recognition system written entirely in the JavaTM programming language. It was created via a joint collaboration between the Sphinx group at Carnegie Mellon University, Sun Microsystems Laboratories, Mitsubishi Electric Research Labs (MERL), and Hewlett Packard (HP), with contributions from the University of California at Santa Cruz (UCSC) and the Massachusetts Institute of Technology (MIT).
Sphinx-4 started out as a port of Sphinx-3 to the Java programming language, but evolved into a recognizer designed to be much more flexible than Sphinx-3, thus becoming an excellent platform for speech research.
1) Download the software:
Here is the download link:
http://sourceforge.net/projects/cmusphinx/files/sphinx4/1.0%20beta6/sphinx4-1.0beta6-bin.zip/download.
2) Create new Java project:
Add to class-path 3 jars from the lib folder:
jsapi.jar (if not exist jsapi.exe will extract it on windows or jsapi.sh will extract it)
sphinx4.jar (APIs Jar)
WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.jar (this is the jar containing the speech)
3) Create a new class to write the logic on it: e.g. ListenToMe.java
Let the package name osa.ora
4) Add the file dict.gram to the same package: osa.ora
It should looks like:
#JSGF V1.0;
grammar hello;
public <greet>
The structure is to write the alternative words between () and separated by | and give them tag name to describe these words (greet here).
The grammar specs in this URL:
http://www.w3.org/TR/jsgf/
A quick tutorial in this URL:
http://puneetk.com/basics-of-java-speech-grammar-format-jsgf
5) Add the config file myconfig.xml to the same package: osa.ora
You can get the file structure from the jar in bin folder HelloWorld.jar
in the location "HelloWorld.jar\edu\cmu\sphinx\demo\helloworld\helloworld.config.xml"
You need to edit the following entry:
<component name="jsgfGrammar" type="edu.cmu.sphinx.jsgf.JSGFGrammar">
<property name="dictionary" value="dictionary"/>
<property name="grammarLocation"
value="resource:/osa/ora/"/>
<property name="grammarName" value="dict"/>
<property name="logMath" value="logMath"/>
</component>
6) Write the following code in the created class:
import edu.cmu.sphinx.frontend.util.Microphone;
import edu.cmu.sphinx.recognizer.Recognizer;
import edu.cmu.sphinx.result.Result;
import edu.cmu.sphinx.util.props.ConfigurationManager;
public static void main(String args[]){
ConfigurationManager cm = new ConfigurationManager(ListenToMe.class.getResource("myconfig.xml"));
Recognizer recognizer = (Recognizer)cm.lookup("recognizer");
recognizer.allocate();
Microphone microphone = (Microphone)cm.lookup("microphone");
if(!microphone.startRecording()){
System.out.println("Cannot start microphone.");
recognizer.deallocate();
System.exit(1);
}
System.out.println("Say: (Good morning | Hello | Hi | Welcome) ( Osama | Paul | Philip | Rita | Will )");
do {
System.out.println("Start speaking. Press Ctrl-C to quit.\n");
Result result = recognizer.recognize();
if(result != null) {
String resultText = result.getBestFinalResultNoFiller();
System.out.println((new StringBuilder()).append("You said: ").append(resultText).append('\n').toString());
} else {
System.out.println("I can't hear what you said.\n");
}
} while(true);
}
As you can see you can use ConfigurationManager to re-set the grammarName to dict1 , dict2 ,..etc, you can also have multiple configurations.
For more details about the usage you can refer to this URL:
http://cmusphinx.sourceforge.net/sphinx4/
You may use Interactive4J implementation direct to achieve dynamic voice recognition.. Find more details here.
Hi,
ReplyDeleteI followed your instructions, but I have an exception:
class not found !java.lang.ClassNotFoundException: edu.cmu.sphinx.model.acoustic.WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.Model
Exception in thread "main" Property exception component:'flatLinguist' property:'acousticModel' - component 'wsj' is missing
How to solve it?
Nice tutorial
Cheers
bren [at] juanantonio.info
This comment has been removed by the author.
ReplyDeleteYou must add this jar to the classpath:
ReplyDeleteWSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.jar
Thanks
I added but I continue with problems.
ReplyDeleteis it possible to change that value or replace?
http://img217.imageshack.us/f/sphinx4.png/
Cheers
Could you send me your configuration files or project, seems there is something with configuration...
ReplyDelete<property name="dictionaryPath"
ReplyDeletevalue="resource:/WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz/dict/cmudict.0.6d"/>
<property name="fillerPath"
value="resource:/WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz/dict/fillerdict"/>
Hi Osama, I solved my problem with your help. My problem was located in configuration file.
ReplyDeleteCheers
We are facing same problem . Could you plz mention what changes you did in config file ?
DeleteHello Sir, i got the same error with edu.I checked the config file.Everything is fine but error is not getting rectified.
DeleteThanks
Dear Osama,
ReplyDeletei tried every thing but it did not work and i think the problem in fillerdict as it is not there in the jar file, can you tell me what to do?
What is the issue or the exception?
ReplyDeletehi ossama:
ReplyDeletei have problem in configuration manager please send this file to me
thanks
what do you mean by configuration manager ? you mean configuration file ?
ReplyDeleteyes osama but this problem have been solved thanks for you
ReplyDeletecan you tell me how can i changes words in grammer if i need this
thanks
Just edit the file using notepad or similar apps and add whatever you want , if any word is unsupported you will see warning.
ReplyDeletesorry i can't understand what do you mean i change words in grammer file and in java class but the output is the same words in helloworld example
ReplyDeleteIn configuration file, you must point to this new file location.
ReplyDelete<property name="grammarLocation"
ReplyDeletevalue="resource:/osa/ora/"/>
<property name="grammarName" value="dict"/>
or use the following location (outside your jar) :
ReplyDelete<property name="grammarLocation"
value="file:///C:/config/"/>
osama i need to add this words to my app(category-next-previous-select- sub category- product-go category-feature product-go first category)
ReplyDeleteplease did you send to me your mail in<azza_az20@yahoo.com)to send to you my application to edit this words
i need this quickly because it is part of graduation project and it will deliver in 21/7 pleas osama help me quickly\
thanks for you
My advice is to keep 1 word for each command : remove "go" for example also remove category so your dictionary looks like : First, Feature, Category, Product, Sub, Select, Next, Previous
ReplyDeleteTry the application and remove the dominant words and replace them with alternatives with the same meaning. like Previous --> Back , Next -->Move ,...etc.. until you get the best results possible.
Hi Osama,
ReplyDeleteThis is a good post.
I have followed all the steps
1. I created a eclipse project
2. Copied the jar files/class files.
3. Execute the project
i get the following error
Exception in thread "main" java.lang.NullPointerException
at osa.ora.ListenToMe.main(ListenToMe.java:12)
For the line number 12, which is
Recognizer recognizer = (Recognizer)cm.lookup("recognizer");
recognizer.allocate();
Could you please help me with this ..
What is ur email id ???
thanks ossama the program is running but what i have to achieve the accuracy
ReplyDeletethanks
Ensure the configurations are correctly in the class-path and all needed jars exist in the class path.
ReplyDeleteWhat if I want to use Arabic words in the grammar
ReplyDeleteThanks
The correct way is to have a new language that support this implementation for Arabic because this current implementation for English, so i advice you to search for another way if you need it quickly as this need a lot of time and effort plus a big team work to develop it.
ReplyDeletehello Mr Osama
ReplyDeleteYou have wrote a great article...
thanks for this
i am trying to run a simple application using sphinx as guided by you in this article and am not able to run the application but got the exception
D:\Sound>java -mx256m osa.ora.ListenToMe
Exception in thread "main" java.lang.RuntimeException: searchManager: allocation
of search manager resources failed
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.allocat
e(SimpleBreadthFirstSearchManager.java:643)
at edu.cmu.sphinx.decoder.AbstractDecoder.allocate(AbstractDecoder.java:
87)
at edu.cmu.sphinx.recognizer.Recognizer.allocate(Recognizer.java:168)
at osa.ora.ListenToMe.main(ListenToMe.java:13)
Caused by: java.io.FileNotFoundException: JAR entry WSJ_8gau_13dCep_16k_40mel_13
0Hz_6800Hz//means not found in D:\API's & Look and Feels\speech data\sphinx4-1.0
beta4-bin\sphinx4-1.0beta4\lib\WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.jar
at sun.net.www.protocol.jar.JarURLConnection.connect(JarURLConnection.ja
va:122)
please help...
or send me a simple ListenToMe project
Thanx.....
The exception said file not found , so either file is not found in the mentioned location or corrupted , you need to include it in your class path , this jar is part of Sphinx4 library:
ReplyDeletejava.io.FileNotFoundException: JAR entry WSJ_8gau_13dCep_16k_40mel_13
0Hz_6800Hz//means not found in D:\API's & Look and Feels\speech data\sphinx4-1.0
beta4-bin\sphinx4-1.0beta4\lib\WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.jar
Hello Mr Osama..
ReplyDeleteThank you for reverting back
I have tried that again but getting the same exception
even i tried to compile and run the Helloworld example provided with sphinx, the file compiles with but fails to run with same exception
the strange thing is that the compile jar(i.e Helloworld.jar) provided with sphinx runs well,
is there any way to get help or running example
Thanks
I guess this is because the Jar exist in the same folder with HelloWorld jar file so it run without any issues.
ReplyDeletehi,
ReplyDeletei have followed all the steps mentioned above.but i'm getting the following error..plz help me
Exception in thread "main" java.lang.RuntimeException: java.io.IOException: Error while parsing line 1 of file:/C:/Program%20Files/Java/speechtotext/sphinx/bin/osa/ora/myconfig.xml: The processing instruction target matching "[xX][mM][lL]" is not allowed.
at edu.cmu.sphinx.util.props.ConfigurationManager.(ConfigurationManager.java:61)
at osa.ora.helloworld.main(helloworld.java:10)
Caused by: java.io.IOException: Error while parsing line 1 of file:/C:/Program%20Files/Java/speechtotext/sphinx/bin/osa/ora/myconfig.xml: The processing instruction target matching "[xX][mM][lL]" is not allowed.
atedu.cmu.sphinx.util.props.SaxLoader.load(SaxLoader.java:77)
at edu.cmu.sphinx.util.props.ConfigurationManager.(ConfigurationManager.java:59)
... 1 more
The exception is clear , your configuration file: myconfig.xml is not valid, please revisit it and if you failed send to me to fix it.
ReplyDeleteHello sir I am getting the following Exceptions:
ReplyDeleteException in thread "main" java.lang.NoSuchFieldError: engineListeners
at com.sun.speech.engine.recognition.BaseRecognizer.fireRecognizerSuspended(BaseRecognizer.java:979)
at com.sun.speech.engine.recognition.BaseRecognizer.dispatchSpeechEvent(BaseRecognizer.java:1272)
at com.sun.speech.engine.SpeechEventUtilities.postSpeechEvent(SpeechEventUtilities.java:201)
at com.sun.speech.engine.SpeechEventUtilities.postSpeechEvent(SpeechEventUtilities.java:132)
at com.sun.speech.engine.recognition.BaseRecognizer.postRecognizerSuspended(BaseRecognizer.java:967)
at com.sun.speech.engine.recognition.BaseRecognizer.commitChanges(BaseRecognizer.java:377)
at edu.cmu.sphinx.jsapi.JSGFGrammar.commitChanges(JSGFGrammar.java:620)
at edu.cmu.sphinx.jsapi.JSGFGrammar.createGrammar(JSGFGrammar.java:318)
at edu.cmu.sphinx.linguist.language.grammar.Grammar.allocate(Grammar.java:163)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.allocate(FlatLinguist.java:319)
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.allocate(SimpleBreadthFirstSearchManager.java:602)
at edu.cmu.sphinx.decoder.Decoder.allocate(Decoder.java:109)
at edu.cmu.sphinx.recognizer.Recognizer.allocate(Recognizer.java:182)
at com.speech.Speech2Text.main(Speech2Text.java:14)
please help me.
Exception in thread "main" "Exception: Allocation of search manager resources failed..."
ReplyDeletePlease Tell me that how to solve it..I have waste two days to solve..but I cannot go forward..My e-mail is iro.amila@gmail.com
This exception "Exception in thread "main" java.lang.NoSuchFieldError: engineListeners" seems to be related to incorrect version used or conflict in your jars... you may use my follow the steps and re-test..
ReplyDeletehello Mr Osama,
ReplyDeleteI checked all configurations, but I'm still getting the following Exceptions:
Exception in thread "main" java.lang.RuntimeException: Allocation of search manager resources failed
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.allocate(SimpleBreadthFirstSearchManager.java:650)
at edu.cmu.sphinx.decoder.AbstractDecoder.allocate(AbstractDecoder.java:87)
at edu.cmu.sphinx.recognizer.Recognizer.allocate(Recognizer.java:168)
at test.helloWorld.main(helloWorld.java:14)
Caused by: java.io.IOException: edu.cmu.sphinx.jsgf.JSGFGrammarParseException
at edu.cmu.sphinx.jsgf.JSGFGrammar.createGrammar(JSGFGrammar.java:304)
at edu.cmu.sphinx.linguist.language.grammar.Grammar.allocate(Grammar.java:116)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.allocate(FlatLinguist.java:300)
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.allocate(SimpleBreadthFirstSearchManager.java:646)
... 3 more
Caused by: edu.cmu.sphinx.jsgf.JSGFGrammarParseException
at edu.cmu.sphinx.jsgf.parser.JSGFParser.newGrammarFromJSGF(JSGFParser.java:132)
at edu.cmu.sphinx.jsgf.parser.JSGFParser.newGrammarFromJSGF(JSGFParser.java:241)
at edu.cmu.sphinx.jsgf.JSGFGrammar.loadNamedGrammar(JSGFGrammar.java:697)
at edu.cmu.sphinx.jsgf.JSGFGrammar.commitChanges(JSGFGrammar.java:613)
at edu.cmu.sphinx.jsgf.JSGFGrammar.createGrammar(JSGFGrammar.java:300)
... 6 more
Thanks a lot.
This seems related to your grammer file, please try to validate its syntax again.
ReplyDeleteYou were right, instead of:
ReplyDelete"public = (Good morning | Hello | Hi | Welcome) ( Osama | Paul | Philip | Rita | Will );"
I used:
"public = (Good morning | Hello | Hi | Welcome) ( Osama | Paul | Philip | Rita | Will );" as in examples.
Thanks a lot.
Same problem :(
DeletePlease tell more how did you solve this one >> thanks
Ok thanks now it is running correctly ..
DeleteThanks Osman
ReplyDeletehello
ReplyDeletethis site is vv v useful
m not finding dict.gram file
wer is it located?
waiting for reply
thanks
You need to create this file yourself using any text editor and locate it in your class package : Here is the sample of this file content:
ReplyDelete#JSGF V1.0;
grammar hello;
public = (Good morning | Hello | Hi | Welcome) ( Osama | Paul | Philip | Rita | Will );
hi i worked this project in netbeans but this error appear :
DeleteException in thread "main" java.lang.NullPointerException
at edu.cmu.sphinx.model.acoustic.WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.ModelLoader.loadProperties(ModelLoader.java:372)
at edu.cmu.sphinx.model.acoustic.WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.ModelLoader.getIsBinaryDefault(ModelLoader.java:386)
at edu.cmu.sphinx.model.acoustic.WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.ModelLoader.newProperties(ModelLoader.java:346)
at edu.cmu.sphinx.util.props.ConfigurationManager.lookup(ConfigurationManager.java:214)
at edu.cmu.sphinx.util.props.ValidatingPropertySheet.getComponent(ValidatingPropertySheet.java:403)
at edu.cmu.sphinx.model.acoustic.WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.Model.newProperties(Model.java:159)
at edu.cmu.sphinx.util.props.ConfigurationManager.lookup(ConfigurationManager.java:214)
at edu.cmu.sphinx.util.props.ValidatingPropertySheet.getComponent(ValidatingPropertySheet.java:403)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.setupAcousticModel(FlatLinguist.java:299)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.newProperties(FlatLinguist.java:246)
at edu.cmu.sphinx.util.props.ConfigurationManager.lookup(ConfigurationManager.java:214)
at edu.cmu.sphinx.util.props.ValidatingPropertySheet.getComponent(ValidatingPropertySheet.java:403)
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.newProperties(SimpleBreadthFirstSearchManager.java:180)
at edu.cmu.sphinx.util.props.ConfigurationManager.lookup(ConfigurationManager.java:214)
at edu.cmu.sphinx.util.props.ValidatingPropertySheet.getComponent(ValidatingPropertySheet.java:403)
at edu.cmu.sphinx.decoder.Decoder.newProperties(Decoder.java:71)
at edu.cmu.sphinx.util.props.ConfigurationManager.lookup(ConfigurationManager.java:214)
at edu.cmu.sphinx.util.props.ValidatingPropertySheet.getComponent(ValidatingPropertySheet.java:403)
at edu.cmu.sphinx.recognizer.Recognizer.newProperties(Recognizer.java:93)
at edu.cmu.sphinx.util.props.ConfigurationManager.lookup(ConfigurationManager.java:214)
at HelloWorld.main(HelloWorld.java:37)
this is line 37:
Recognizer recognizer = (Recognizer) cm.lookup("recognizer");
Please refer to step 5.
DeleteYou need to add the WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.jar file as a project dependency.
DeleteIn Eclipse, in the pom.xml file, I added:
cmu.sphinx
WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz
4.0
Then, I did a local Maven install from Git Bash command line:
mvn install:install-file -Dfile="/Users/sizu/Desktop/sphinx4-1.0beta6-bin/sphinx4-1.0beta6/lib/WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.jar" -DgroupId="cmu.sphinx" -DartifactId="WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz" -Dversion="4.0" -Dpackaging="jar"
hi Osama
ReplyDeleteI worked on the project in netbeans but always error appear on this line :
Recognizer recognizer = (Recognizer) cm.lookup("recognizer");
how I fix that ?
Please refer to step 5.
DeleteBut I add the xml file to the same path of the java file
Delete!!!
and i adjust the path in the project :
url = new File("C:\\Users\\pc2020\\Desktop\\New folder\\sphinx4-1.0beta\\bin\\HelloWorld.jar\\edu\\cmu\\sphinx\\demo\\helloworld\\helloworld.config.xml").toURI().toURL();
but i add the xml file into the same path into the path of java file :
Deleteurl = new File("C:\\Users\\pc2020\\JavaApplication10\\src\\e.xml").toURI().toURL();
Just add the file to the same location of your path, and do the needed modifications :
ReplyDelete<property name="grammarLocation"
value="resource:/osa/ora/"/>
<property name="grammarName" value="dict"/>
sorry can you write the modification according to my location
ReplyDeleteDid you mean:
or what !!!
hi again
ReplyDeleteI've done all the steps again as it is written, but still error is???
please help me this is important step in my project?
send me your eamil / or your Netbeans project to fix the issue.
DeleteI examined your work , the issue is that you have named the grammer as hello.gram , while in configuration file it is dict.gram:
Delete<property name="grammarName" value="dict"/>
change this into:
<property name="grammarName" value="hello"/>
xception in thread "main" java.lang.OutOfMemoryError: Java heap space
ReplyDeleteat java.lang.String.toLowerCase(String.java:2474)
at java.lang.String.toLowerCase(String.java:2497)
at edu.cmu.sphinx.linguist.dictionary.FastDictionary.loadDictionary(FastDictionary.java:259)
at edu.cmu.sphinx.linguist.dictionary.FastDictionary.allocate(FastDictionary.java:198)
at edu.cmu.sphinx.linguist.language.grammar.Grammar.allocate(Grammar.java:112)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.allocate(FlatLinguist.java:300)
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.allocate(SimpleBreadthFirstSearchManager.java:646)
at edu.cmu.sphinx.decoder.AbstractDecoder.allocate(AbstractDecoder.java:87)
at edu.cmu.sphinx.recognizer.Recognizer.allocate(Recognizer.java:168)
at speechrecog.ListenToMe.main(ListenToMe.java:20)
Seems memeory issue, can you try again and take memory dump and analyze it... can u send me ur project?
Deletehello Osama,
ReplyDeleteCan I use Sphinx engine for languages with non latin alphabets like Arabic? Thanks
hello Osama i am actually working over a project "voice controlled desktop " which involves speech recognition but i am facing this very strange problem : "Can't open microphone line with format PCM_SIGNED 16000.0 Hz, 16 bit, mono, 2 bytes/frame, big-endian not supported."
ReplyDeletethis error appears when i navigate from one page to another in my project . Could u help me to solve this problem
Seems you need to close it before navigating to the other pages then re-open it again.
DeleteThanx for ur reply . but i am already doing this by using command Recognizer.deallocate() but still this error is appearing. may be i m missing some steps
Deletecould u explain me in steps using sphinx commands how should i navigate from one page to another it will be great help to me.
Can you have a look on my interactive4J application, i did use it without any issues, you may download the code and see my wrapper and the demo?
DeleteThanx a lot ! this project of urs will be great help to me. I must say u are truly genius i just went through all ur projects they are so much authentic and full of innovation & intelligence .
ReplyDeleteThanks a lot for your nice words..
DeleteHello Osama!! i m being able to run in netbeans bt the output is running in the console as its a .java file...how can i mk it run in the browser?
ReplyDeletewhat browser ? you can check interactive browser one of my open source projects to see how you can do this ...
DeleteThnx 4 the reply...wl check out ur open source projct...n i m talking abt browsers like internet explorer,google chrome,firefox etc...actually i m working on a project that will conduct examination 4 da blind people...sphinx-4 helped me in the conversion from speech 2 text.bt at times it cudn't recognise correctly(like 'four' s recognised as 'two').cn u gv me any suggestions to rectify dt? n also i hv 2 mk it work in da browser.
DeleteHello, Can you help me fix the config file(Netbean project)
ReplyDeletethank you for your reply.
http://www.mediafire.com/?evq06u94j5qafq1
Maybe I know what is the problems about the .xml file ?
DeleteIf I follow your example above, it should be the path location causes the null pointer exception. thanks in advance.
May I know what is the problem for the netbean project? I'm think it's the .xml file can't find the location path. If I follow the example above, it causes null pointer exception, I'm think the problems occurred in .xml file because the path is not defined correctly. thanks in advance.
DeleteI checked your file, the path is invalid and the filename as well ..
Deleteresource:file:C\\Users\\User\\Documents\\NetBeansProjects\\osa.ora\\
the prefix resource: means it is a resource inside the jar so use relative path as in my example, in your project you didn't define a package for your java classes (this is not good) any way the value should be in that case resource:/
the filename in your example is : hello.gram so the other value:
<property name="grammarName" value="hello"/>
Hello Osama Oransa, thank for your help and explaination, now I can run my sample program.
DeleteHi Osama,
ReplyDeleteits an amazing example to start with.
can you please mail or post an example of dictation grammar.
please it will be of great help
Please clarify what you mean ? an example of grammer file is already in the post.
DeleteHi osama!great tutorial!
ReplyDeleteI have a problem: I followed all the steps of the tutorial and I have no errors in compilation, but the program remains on after being launched Say: (Good morning | Hello | Hi | Welcome) (Usama | Paul | Philip | Rita | Will)
Start speaking. Press Ctrl-C to quit.
and then nothing happens .. why?
I guess this is a mic problem, try to speek these words until it recognizes any of them..
DeleteHi osama, i have this problem
ReplyDeleteException in thread "main" java.lang.RuntimeException: Allocation of search manager resources failed
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.allocate(SimpleBreadthFirstSearchManager.java:650)
at edu.cmu.sphinx.decoder.AbstractDecoder.allocate(AbstractDecoder.java:87)
at edu.cmu.sphinx.recognizer.Recognizer.allocate(Recognizer.java:168)
at osa.ora.ListenToMe.main(ListenToMe.java:13)
Caused by: java.io.IOException: edu.cmu.sphinx.jsgf.JSGFGrammarParseException
at edu.cmu.sphinx.jsgf.JSGFGrammar.createGrammar(JSGFGrammar.java:304)
at edu.cmu.sphinx.linguist.language.grammar.Grammar.allocate(Grammar.java:116)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.allocate(FlatLinguist.java:300)
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.allocate(SimpleBreadthFirstSearchManager.java:646)
... 3 more
Caused by: edu.cmu.sphinx.jsgf.JSGFGrammarParseException
at edu.cmu.sphinx.jsgf.parser.JSGFParser.newGrammarFromJSGF(JSGFParser.java:132)
at edu.cmu.sphinx.jsgf.parser.JSGFParser.newGrammarFromJSGF(JSGFParser.java:241)
at edu.cmu.sphinx.jsgf.JSGFGrammar.loadNamedGrammar(JSGFGrammar.java:697)
at edu.cmu.sphinx.jsgf.JSGFGrammar.commitChanges(JSGFGrammar.java:613)
at edu.cmu.sphinx.jsgf.JSGFGrammar.createGrammar(JSGFGrammar.java:300)
... 6 more
i checked grammar, ctrl c + ctr v in your sample, checked config.xml
thanks for your help!
This is grammar syntax error , try to fix your grammar , may be special character exist.
ReplyDeletehi osama i got the following error:
ReplyDeleteSyntax error on token "void", @ expected
Syntax error on token "String", instanceof expected after this token
Syntax error, insert "enum Identifier" to complete EnumHeader
at osa.ora.ListenToMe.main(ListenToMe.java:11)
here is what i tried:
package osa.ora;
public class ListenToMe
{
import edu.cmu.sphinx.frontend.util.Microphone;
import edu.cmu.sphinx.recognizer.Recognizer;
import edu.cmu.sphinx.result.Result;
import edu.cmu.sphinx.util.props.ConfigurationManager;
public static void main(String args[]){
ConfigurationManager cm = new ConfigurationManager(ListenToMe.class.getResource("myconfig.xml"));
Recognizer recognizer = (Recognizer)cm.lookup("recognizer");
recognizer.allocate();
Microphone microphone = (Microphone)cm.lookup("microphone");
if(!microphone.startRecording()){
System.out.println("Cannot start microphone.");
recognizer.deallocate();
System.exit(1);
}
System.out.println("Say: (Good morning | Hello | Hi | Welcome) ( Osama | Paul | Philip | Rita | Will )");
do {
System.out.println("Start speaking. Press Ctrl-C to quit.\n");
Result result = recognizer.recognize();
if(result != null) {
String resultText = result.getBestFinalResultNoFiller();
System.out.println((new StringBuilder()).append("You said: ").append(resultText).append('\n').toString());
} else {
System.out.println("I can't hear what you said.\n");
}
} while(true);
}
}
plz help me
Ensure your imports are complete , here is mine for example:
Deleteimport edu.cmu.sphinx.frontend.util.Microphone;
import edu.cmu.sphinx.recognizer.Recognizer;
import edu.cmu.sphinx.result.Result;
import edu.cmu.sphinx.util.props.ConfigurationManager;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.OutputStreamWriter;
import java.util.Enumeration;
import java.util.Hashtable;
i added all the imports you mentioned still got the same error
ReplyDeletewhat could i do?
What is the exception now ?
DeleteSyntax error on token "void", @ expected
DeleteSyntax error on token "String", instanceof expected after this token
Syntax error, insert "enum Identifier" to complete EnumHeader
at osa.ora.ListenToMe.main(ListenToMe.java:11)
It seems you are new to Java ?
DeleteThis is a compilation errors i can't help with , you need to fix them.
Hi osama i want to create a voice recognizer by import javax.speech.recognition package. will you help me on this. i have only ive days..... please help me. thanks in advence.....
ReplyDeleteOk, what you want exactly ? you may follow the post steps or other open source projects?
DeleteHello osama... i am a java beginner.... thanks for your article... it was a lot helpful... i started working on it.... but now i am facing a problem...
ReplyDeleteException in thread "main" java.lang.NullPointerException
//at : recognizer.allocate();
can u plz help me
Actually i remember null pointer could means the WebCam USB is not connected or not working properly (may be the driver is not installed)..Otherwise please post the whole exception stacktrack or look in the previous comments for a resolution.
Deleteyes my microphone is properly inserted...
ReplyDeletemyconfig.xml file is
//package name is helloworld
shall i need to write anything in the ListenToMe() ???
if yes give me a sample......
thanks in advance
I don't see any file content here ?
Deleteis it mandatory to connect the mic on frontend???
ReplyDeletehi Osama .. first thanks for this good job
ReplyDeleteso .. i did exactly the way to told us to do .. but i got some errors .. can i send you my work to see it
hello Osama
ReplyDeletei followed all the steps but i have a exception
Exception in thread "main" java.lang.NullPointerException
at edu.cmu.sphinx.util.props.SaxLoader.load(SaxLoader.java:74)
at edu.cmu.sphinx.util.props.ConfigurationManager.(ConfigurationManager.java:58)
at ListenToMe.main(ListenToMe.java:10)
and i read all the comment but i can't fix my problem voice recognition is a part of my project so please help me
The configuration file is not configured correctly, please ensure to place it in the correct path.
Deletedear osama
ReplyDeletei m confused with myconfig.xml file so can tell editing again clearly
thanxx
dear osama
ReplyDeletei m confused with myconfig.xml file so can tell editing again clearly
thanxx
dear osama
ReplyDeletei am confused with myconfig.xml as where to edit so can u please help me out
hiii these are my errorat console
ReplyDeletei m using eclipse and have added all jars ,dict,myconfig file
plz help me out
Exception in thread "main" java.lang.RuntimeException: Allocation of search manager resources failed
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.allocate(SimpleBreadthFirstSearchManager.java:650)
at edu.cmu.sphinx.decoder.AbstractDecoder.allocate(AbstractDecoder.java:87)
at edu.cmu.sphinx.recognizer.Recognizer.allocate(Recognizer.java:168)
at pkg.ListenToMe.main(ListenToMe.java:17)
Caused by: java.io.IOException: edu.cmu.sphinx.jsgf.JSGFGrammarParseException
at edu.cmu.sphinx.jsgf.JSGFGrammar.createGrammar(JSGFGrammar.java:304)
at edu.cmu.sphinx.linguist.language.grammar.Grammar.allocate(Grammar.java:116)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.allocate(FlatLinguist.java:300)
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.allocate(SimpleBreadthFirstSearchManager.java:646)
... 3 more
Caused by: edu.cmu.sphinx.jsgf.JSGFGrammarParseException
at edu.cmu.sphinx.jsgf.parser.JSGFParser.newGrammarFromJSGF(JSGFParser.java:132)
at edu.cmu.sphinx.jsgf.parser.JSGFParser.newGrammarFromJSGF(JSGFParser.java:241)
at edu.cmu.sphinx.jsgf.JSGFGrammar.loadNamedGrammar(JSGFGrammar.java:697)
at edu.cmu.sphinx.jsgf.JSGFGrammar.commitChanges(JSGFGrammar.java:613)
at edu.cmu.sphinx.jsgf.JSGFGrammar.createGrammar(JSGFGrammar.java:300)
... 6 more
The grammer file is invalid or not available at the specified path.
Deletehi i have followed all the steps but i have following error
ReplyDelete12:11:17.689 SEVERE wsj Can't find HMM for EI
Exception in thread "main" java.lang.NullPointerException
at edu.cmu.sphinx.linguist.flat.FlatLinguist$GState.getHMMStates(FlatLinguist.java:1216)
at edu.cmu.sphinx.linguist.flat.FlatLinguist$GState.expandUnit(FlatLinguist.java:1193)
at edu.cmu.sphinx.linguist.flat.FlatLinguist$GState.attachUnit(FlatLinguist.java:1041)
at edu.cmu.sphinx.linguist.flat.FlatLinguist$GState.expandPronunciation(FlatLinguist.java:992)
at edu.cmu.sphinx.linguist.flat.FlatLinguist$GState.expandWord(FlatLinguist.java:912)
at edu.cmu.sphinx.linguist.flat.FlatLinguist$GState.expand(FlatLinguist.java:825)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.compileGrammar(FlatLinguist.java:420)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.allocate(FlatLinguist.java:304)
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.allocate(SimpleBreadthFirstSearchManager.java:646)
at edu.cmu.sphinx.decoder.AbstractDecoder.allocate(AbstractDecoder.java:87)
at edu.cmu.sphinx.recognizer.Recognizer.allocate(Recognizer.java:168)
at Experiment.Speech.ListenToMe.main(ListenToMe.java:14)
Line no. 14 is recognizer.allocate();
Plz help
Seems there is an issue with your grammer file, can you re-check it again.
DeleteSalam Osama!
ReplyDeletei need ur help in my final year project which is based on speech recognition. i didn't get ur step num 2 and 4 kindly help me how to add jar files in class path? If possible so plz send me ur id actually we r making virtual Agent plz help us
Can you download interactive4J and u will find all the needed configurations, please let me know if you still need any help.
DeleteDownload the source code to see how you need to write this, currently you have downloaded the jar file.
Deletei have downloaded interactive4j. it is a jar file now plz tell me how to use this file in IDE (Eclipse).
ReplyDeleteHello Sir
ReplyDeleteI am getting the following Exception
run:
Loading...
Problem configuring HelloWorld: Property exception component:'jsgfGrammar' property:'grammarLocation' - Can't locate resource:C:\\Users\\vaibhav\\Documents\\NetBeansProjects\\sphinxdemo\\src
edu.cmu.sphinx.util.props.InternalConfigurationException: Can't locate resource:C:\\Users\\vaibhav\\Documents\\NetBeansProjects\\sphinxdemo\\src
Property exception component:'jsgfGrammar' property:'grammarLocation' - Can't locate resource:C:\\Users\\vaibhav\\Documents\\NetBeansProjects\\sphinxdemo\\src
edu.cmu.sphinx.util.props.InternalConfigurationException: Can't locate resource:C:\\Users\\vaibhav\\Documents\\NetBeansProjects\\sphinxdemo\\src
at edu.cmu.sphinx.util.props.ConfigurationManagerUtils.getResource(ConfigurationManagerUtils.java:483)
at edu.cmu.sphinx.jsgf.JSGFGrammar.newProperties(JSGFGrammar.java:232)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.newProperties(FlatLinguist.java:246)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.newProperties(SimpleBreadthFirstSearchManager.java:182)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.decoder.AbstractDecoder.newProperties(AbstractDecoder.java:65)
at edu.cmu.sphinx.decoder.Decoder.newProperties(Decoder.java:37)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.recognizer.Recognizer.newProperties(Recognizer.java:90)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.ConfigurationManager.lookup(ConfigurationManager.java:161)
at HelloWorld.main(HelloWorld.java:50)
BUILD SUCCESSFUL (total time: 3 seconds)
Please sir i am in great need of help of your's. How could i deal with this ?
component:'jsgfGrammar' property:'grammarLocation' - Can't locate resource:C:\\Users\\vaibhav\\Documents\\NetBeansProjects\\sphinxdemo\\src
ReplyDeleteThis is from the exception , you should see what is wrong in grammer location config file.
SOLUTION:
ReplyDeleteThe problem here for most of you is the grammar line:
public = (...
You think it's "public = (...". It's not.
There is a "greet" element after the word public.
It can't be seen (on Firefox, at least).
However, you can see it if you right click on the browser and view the page source.
This is the actual code: public <greet> = (...
Thanks I have fixed it using <
DeleteI have fixed it...thanks for nice article.
ReplyDeleteRegards-
Vikalp Sharma.
I have fixed it...thanks for nice article.
ReplyDeleteRegards-
Vikalp Sharma.
Hi,
ReplyDeleteI want sphinx to be executed again and again.if i put in loop with
reinitialising recogniser and microphone...,it works....But wen i try to reinitialise the Configuration Manager again,it gives the following exception
09:45:32.124 SEVERE microphone Can't open microphone mummy line with format PCM_SIGNED 16000.0 Hz, 16 bit, mono, 2 bytes/frame, big-endian not supported.
could u help me with clearing this pls?
Hi,
ReplyDeleteafter following above 6 steps I got error
Exception in thread "main" java.lang.NullPointerException
at edu.cmu.sphinx.util.props.SaxLoader.load(SaxLoader.java:74)
at edu.cmu.sphinx.util.props.ConfigurationManager.(ConfigurationManager.java:58)
at osa.ora.ListenToMe.main(ListenToMe.java:17)
Suresh :
ReplyDeleteHi,
after following above 6 steps I got error
Exception in thread "main" java.lang.NullPointerException
at edu.cmu.sphinx.util.props.SaxLoader.load(SaxLoader.java:74)
at edu.cmu.sphinx.util.props.ConfigurationManager.(ConfigurationManager.java:58)
at osa.ora.ListenToMe.main(ListenToMe.java:17)
Osama: This is configuration file issue, fix it ...
hai,
ReplyDeletei followed the steps what u have listed.
still i need to know to change grammar location.
And when i run i have some errors.
Exception in thread "main" Property exception component:'jsgfGrammar' property:'grammarLocation' - Can't locate resource:C:/Documents and Settings/user/My Documents/NetBeansProjects/osp/src/osp/
edu.cmu.sphinx.util.props.InternalConfigurationException: Can't locate resource:C:/Documents and Settings/user/My Documents/NetBeansProjects/osp/src/osp/
at edu.cmu.sphinx.util.props.ConfigurationManagerUtils.getResource(ConfigurationManagerUtils.java:483)
at edu.cmu.sphinx.jsgf.JSGFGrammar.newProperties(JSGFGrammar.java:232)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.newProperties(FlatLinguist.java:246)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.newProperties(SimpleBreadthFirstSearchManager.java:182)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.decoder.AbstractDecoder.newProperties(AbstractDecoder.java:65)
at edu.cmu.sphinx.decoder.Decoder.newProperties(Decoder.java:37)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.recognizer.Recognizer.newProperties(Recognizer.java:90)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.ConfigurationManager.lookup(ConfigurationManager.java:161)
at osp.Main.main(Main.java:33)
Java Result: 1
BUILD SUCCESSFUL (total time: 0 seconds)
in some cases i get this type of errors
class not found !java.lang.ClassNotFoundException: edu.cmu.sphinx.model.acoustic.WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.Model
Exception in thread "main" Property exception component:'flatLinguist' property:'acousticModel' - component 'wsj' is missing
edu.cmu.sphinx.util.props.InternalConfigurationException: component 'wsj' is missing
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:289)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.setupAcousticModel(FlatLinguist.java:278)
at edu.cmu.sphinx.linguist.flat.FlatLinguist.newProperties(FlatLinguist.java:244)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.decoder.search.SimpleBreadthFirstSearchManager.newProperties(SimpleBreadthFirstSearchManager.java:182)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.decoder.AbstractDecoder.newProperties(AbstractDecoder.java:65)
at edu.cmu.sphinx.decoder.Decoder.newProperties(Decoder.java:37)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.PropertySheet.getComponent(PropertySheet.java:287)
at edu.cmu.sphinx.recognizer.Recognizer.newProperties(Recognizer.java:90)
at edu.cmu.sphinx.util.props.PropertySheet.getOwner(PropertySheet.java:505)
at edu.cmu.sphinx.util.props.ConfigurationManager.lookup(ConfigurationManager.java:161)
at sphinxttest.Main.main(Main.java:34)
Java Result: 1
BUILD SUCCESSFUL (total time: 0 seconds)
can u explain me what may be the mistakes to be corrected
Please use Interactive4J as a ready example for such functionality with source code available ....
ReplyDeletesalam Alekom
ReplyDeletedoes this method guranteed for arabic language ???
what about using audio fingerprinting??
Wa 3alekom el salam,
DeleteYou need to implement the Arabic language recognition...
It is not supporting audio fingerprinting....
where can i find out "HelloWorld.jar\edu\cmu\sphinx\demo\helloworld\helloworld.config.xml"?
ReplyDeleteis by sphinx4-1.0beta6->bin->helloworld? but that jar is not showing any thing. how can i clarify this problem?
Hi Osama,
ReplyDeleteI am working on voice recognition. I got this error:
Exception in thread "AWT-EventQueue-0" java.lang.Error: Error loading word: About.class
at edu.cmu.sphinx.linguist.dictionary.FastDictionary.loadDictionary(FastDictionary.java:278)
at edu.cmu.sphinx.linguist.dictionary.FastDictionary.allocate(FastDictionary.java:220)
at edu.cmu.sphinx.linguist.lextree.LexTreeLinguist.allocate(LexTreeLinguist.java:340)
at edu.cmu.sphinx.decoder.search.WordPruningBreadthFirstSearchManager.allocate(WordPruningBreadthFirstSearchManager.java:238)
at edu.cmu.sphinx.decoder.AbstractDecoder.allocate(AbstractDecoder.java:87)
at edu.cmu.sphinx.recognizer.Recognizer.allocate(Recognizer.java:168)
at osa.ora.SimpleBrowser.enableVoiceStart(SimpleBrowser.java:351)
at osa.ora.SimpleBrowser.enableVoiceActionPerformed(SimpleBrowser.java:324)
at osa.ora.SimpleBrowser.(SimpleBrowser.java:70)
at osa.ora.SimpleBrowser$11.run(SimpleBrowser.java:376)
at java.awt.event.InvocationEvent.dispatch(InvocationEvent.java:209)
at java.awt.EventQueue.dispatchEvent(EventQueue.java:597)
at java.awt.EventDispatchThread.pumpOneEventForFilters(EventDispatchThread.java:269)
at java.awt.EventDispatchThread.pumpEventsForFilter(EventDispatchThread.java:184)
at java.awt.EventDispatchThread.pumpEventsForHierarchy(EventDispatchThread.java:174)
at java.awt.EventDispatchThread.pumpEvents(EventDispatchThread.java:169)
at java.awt.EventDispatchThread.pumpEvents(EventDispatchThread.java:161)
at java.awt.EventDispatchThread.run(EventDispatchThread.java:122)
BUILD STOPPED (total time: 22 seconds)
I followed your instructions and have do finish with all the things,,, programe is running successfully but further there no progress.
ReplyDeleteYou can download Interactive4J which do the steps in simple way or Interactive Browser app.
Deletedebug:
ReplyDeleteException in thread "main" java.lang.RuntimeException: java.io.IOException: Error while parsing line 14 of file:/C:/Users/User/Documents/NetBeansProjects/GodHelpMe/build/classes/godhelpme/myconfig.xml: The markup in the document following the root element must be well-formed.
at edu.cmu.sphinx.util.props.ConfigurationManager.(ConfigurationManager.java:60)
at godhelpme.GodHelpMe.main(GodHelpMe.java:21)
Caused by: java.io.IOException: Error while parsing line 14 of file:/C:/Users/User/Documents/NetBeansProjects/GodHelpMe/build/classes/godhelpme/myconfig.xml: The markup in the document following the root element must be well-formed.
at edu.cmu.sphinx.util.props.SaxLoader.load(SaxLoader.java:79)
at edu.cmu.sphinx.util.props.ConfigurationManager.(ConfigurationManager.java:58)
... 1 more
Java Result: 1
BUILD SUCCESSFUL (total time: 0 seconds)
The xml file "godhelpme/myconfig.xml" is not correct, re-create it or open it using IE and see if it open well or not.
Deletehi Osama, i am working on sphinx4 i was completed the first program working on MFCC feature vector with help of helloworld.java and WaveFile.java.
ReplyDeletenow i want to do the same program with other feature vector like PLP, LPC or ENH-MFCC).
plz is the change in confi.xml file is enough or i need something else
Sorry Hussein but I have no idea if you can do this..
Deleteaoa sir i m new in java and my project is voice recognition email system in java.i found sphinx4 but it only works on predefined words i have searched enough but i could not find more.i m stuck at that point.if you can help then plz do.i require an API that convert whole english language to text.we are working in java.
ReplyDeleteYes, it need a dictionary for that (predefined word file) ...
ReplyDeleteYou can find other tools here :
http://ocvolume.sourceforge.net/links.php
hello sir will you like to help me in this area??
ReplyDeletei got this error.
09:50.450 WARNING dictionary Missing word: username
in edu.cmu.sphinx.linguist.dictionary.FastDictionary:getWord-dictionary
09:50.450 WARNING jsgfGrammar Can't find pronunciation for username
in edu.cmu.sphinx.linguist.language.grammar.Grammar:createGrammarNode-jsgfGrammar
09:50.450 WARNING dictionary Missing word: login
in edu.cmu.sphinx.linguist.dictionary.FastDictionary:getWord-dictionary
09:50.450 WARNING jsgfGrammar Can't find pronunciation for login
in edu.cmu.sphinx.linguist.language.grammar.Grammar:createGrammarNode-jsgfGrammar
(help | username | password | login | compose | to | subject | body | send | read );
Start speaking. Press Ctrl-C to quit.
Please use "user name" instead of "username" in your dictionary.
Deletewhat about login??
DeleteI think it should be "Log in"
Deletesir can i use speech recognizer and synthesizer both in one application?
DeleteIf you mean text to speech, yes you can find a library to do that.
Deletethank you sir..!
Deleteand can i recognize every word which are spoken??
bcz i need this operation for my application.
Speech recognition will remain area for research for a while, now Google and others have very mature engines for that.
DeleteHello Osama
ReplyDeletemy project depends on the dialog demo that's found in sphinx4-1.0beta6 and am trying to improve the accuracy in recognizing words by loading a new acoustic model that is available :
cmusphinx-en-us-5.2.tar.gz
but failed to load it and I discovered that the new acoustic model has different parameters from the original one with sphinx4-1.0beta6 , Is there a way to load it as I need to improve the accuracy and work on this same version of sphinx4 ??
I appreciate your help.
I'm afraid I can't help on this.
DeleteHi Osama,
ReplyDeleteI using Netbean IDE and i have a error: Can't located resource: /WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz
I add .jar to library. This is classpath ?
Please guide me add WSJ_8gau_13dCep_16k_40mel_130Hz_6800Hz.jar to classpath if you know.
Thank you very much!
Please use Interactive4J as a ready example for such functionality with source code available ....
DeleteException in thread "main" java.lang.NoSuchFieldError: engineListeners
ReplyDeletei'm getting this exception when i'm trying to run sphinx4 project using netbeans can you please help me out
Not sure what is the issue, but review the configurations again and check the library is the one of the system.
Delete