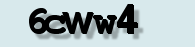
In this post we will learn how to use simple Captcha with Struts to create Captcha for confirming that the interacting user is a person not a computer..
Here is a 5-steps implementation:
1.Download the Simple Captcha jar:
Here is the link:
http://sourceforge.net/projects/simplecaptcha/files/SimpleCaptcha/1.1.1/SimpleCaptcha-1.1.1.jar/download
2.Add the jar to your web project classpath:
Typically inside web-inf/lib folder
3.Add new servlet to your web project:
Name: CaptchaServlet
*Implement doGet method:
try {
Captcha captcha = new Captcha.Builder(200, 50).addText(new DefaultTextProducer(6)).gimp(new DropShadowGimpyRenderer()).build();
//200, 50 is the size, 6 is the number of letters to use, we choose the shadow renderer.
response.setHeader("Cache-Control", "no-store");
response.setHeader("Pragma", "no-cache");
response.setDateHeader("Expires", 0);
// don't cache it , don't store it, expire after 0 second, so with refresh new image loaded
response.setContentType("image/jpeg");
//image type
CaptchaServletUtil.writeImage(response, captcha.getImage());
//write the image
request.getSession().setAttribute("CorrectAnswer",
//store the answer for this in session
} catch (Exception e) {
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR);
return;
}
4.In Your action class: (where the html form that include Captcha is submitted) add the following code:
//registerFormBean is the form bean of the current action and captcha response is the field of user input
String answer =
ActionErrors errors = new ActionErrors();
errors.add("error1", new ActionMessage("error.captcha"));
}
error.captcha=Invalid value of shown text!
//so we check either pass successfully or add to errors error regarding captcha.
5.In the JSP file , add the following:
<img id="captchaImg" src="/...context..../CaptchaServlet" alt="Captcha Image" height="45">
<img src="/...context..../images/reload.jpg" alt="Reload" onclick="document.forms[0].captchaImg.src='/...context..../CaptchaServlet?id='+Math.random();" style="cursor:pointer"/>
<br><font color=red>*</font>Enter Value Shown:
<br><html:errors property="error1"/><br>
<html:text property="captchaResponse" maxlength="6" style="width:100pt"/>
-This will construct the Captcha and the text field to enter the value and struts error message to show errors in captcha response, plus the refresh icon.

NOTE: a good trick we used here is the java script Math.random() function , this way prevent certain browsers like Firefox 3.0 to cache the URL and keep posting the same Captcha image , this enforce it to get the new value without doing any more effort.
Here is how it will looks like:
For more details refer to the web site :
http://simplecaptcha.sourceforge.net/index.html